帮我用C++写一个基于QT的音乐播放器
时间: 2023-04-09 22:01:49 浏览: 139
好的,我可以回答这个问题。首先,您需要安装QT开发环境,并且了解C语言的基本语法。然后,您可以使用QT提供的音频播放器组件来实现音乐播放器的功能。您可以使用QT的QMediaPlayer类来控制音频的播放、暂停、停止等操作,并且可以使用QMediaPlaylist类来管理音频播放列表。在实现过程中,您需要注意处理音频文件的格式、路径等问题,以确保音频可以正常播放。希望这些信息能对您有所帮助。
相关问题
帮我写一个基于C++ 和QT的音乐播放器
好的,我可以回答这个问题。基于C和QT的音乐播放器可以使用QT的多媒体框架来实现,可以使用C语言来编写底层的音频处理和播放功能。您可以使用QT Creator来创建一个新的QT项目,并在其中添加多媒体框架和C语言代码来实现音乐播放器的功能。具体实现细节可以参考QT的官方文档和相关的开源项目。
帮我写一个基于C++和QT的音乐播放器
好的,我可以回答这个问题。下面是一个基于C和QT的音乐播放器的示例代码:
```
#include <QtGui>
#include <QtWidgets>
#include <QtCore>
#include <phonon>
class MusicPlayer : public QWidget
{
Q_OBJECT
public:
MusicPlayer(QWidget *parent = 0);
private slots:
void openFile();
void play();
void pause();
void stop();
void seek(int seconds);
void updatePosition(qint64 position);
void updateDuration(qint64 duration);
private:
QMediaPlayer *player;
QSlider *positionSlider;
QLabel *positionLabel;
QLabel *durationLabel;
QPushButton *playButton;
QPushButton *pauseButton;
QPushButton *stopButton;
};
MusicPlayer::MusicPlayer(QWidget *parent)
: QWidget(parent)
{
QGridLayout *layout = new QGridLayout(this);
positionSlider = new QSlider(Qt::Horizontal, this);
positionSlider->setRange(0, 0);
positionSlider->setToolTip(tr("Position"));
connect(positionSlider, SIGNAL(sliderMoved(int)), this, SLOT(seek(int)));
layout->addWidget(positionSlider, 0, 0, 1, 3);
positionLabel = new QLabel(this);
positionLabel->setMinimumWidth(50);
positionLabel->setText("00:00");
layout->addWidget(positionLabel, 1, 0);
durationLabel = new QLabel(this);
durationLabel->setMinimumWidth(50);
durationLabel->setText("00:00");
layout->addWidget(durationLabel, 1, 2);
playButton = new QPushButton(tr("Play"), this);
connect(playButton, SIGNAL(clicked()), this, SLOT(play()));
layout->addWidget(playButton, 2, 0);
pauseButton = new QPushButton(tr("Pause"), this);
connect(pauseButton, SIGNAL(clicked()), this, SLOT(pause()));
layout->addWidget(pauseButton, 2, 1);
stopButton = new QPushButton(tr("Stop"), this);
connect(stopButton, SIGNAL(clicked()), this, SLOT(stop()));
layout->addWidget(stopButton, 2, 2);
player = new QMediaPlayer(this);
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(updatePosition(qint64)));
connect(player, SIGNAL(durationChanged(qint64)), this, SLOT(updateDuration(qint64)));
}
void MusicPlayer::openFile()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open File"), QDir::homePath());
if (!fileName.isEmpty()) {
player->setMedia(QUrl::fromLocalFile(fileName));
playButton->setEnabled(true);
durationLabel->setText(QString::fromStdString(std::to_string(player->duration() / 1000 / 60) + ":" + std::to_string(player->duration() / 1000 % 60)));
}
}
void MusicPlayer::play()
{
player->play();
playButton->setEnabled(false);
pauseButton->setEnabled(true);
stopButton->setEnabled(true);
}
void MusicPlayer::pause()
{
player->pause();
playButton->setEnabled(true);
pauseButton->setEnabled(false);
}
void MusicPlayer::stop()
{
player->stop();
playButton->setEnabled(true);
pauseButton->setEnabled(false);
stopButton->setEnabled(false);
positionSlider->setValue(0);
}
void MusicPlayer::seek(int seconds)
{
player->setPosition(seconds * 1000);
}
void MusicPlayer::updatePosition(qint64 position)
{
positionLabel->setText(QString::fromStdString(std::to_string(position
阅读全文
相关推荐
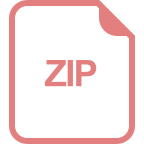
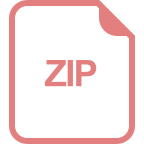
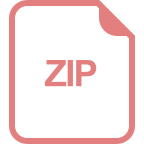













