1. 在IDEA中,使用JDBC对stu表进行如下操作: 添加一条记录: id = 4,name = 赵六,age = 37。 修改记录:将王五的年纪修改为88岁。 删除一条记录:删除李四所在行的记录。定义Stu类 定义方法 public List<Stu> findAll(){} 实现方法 select * from stu;
时间: 2024-02-19 14:58:12 浏览: 33
好的,针对您的问题,我可以给出以下代码:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class Stu {
private int id;
private String name;
private int age;
public Stu(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static void main(String[] args) {
// JDBC连接信息
String driver = "com.mysql.jdbc.Driver";
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "root";
Connection conn = null;
try {
// 加载JDBC驱动
Class.forName(driver);
// 建立连接
conn = DriverManager.getConnection(url, username, password);
// 添加记录
PreparedStatement ps = conn.prepareStatement("insert into stu(id,name,age) values(?,?,?)");
ps.setInt(1, 4);
ps.setString(2, "赵六");
ps.setInt(3, 37);
ps.executeUpdate();
// 修改记录
ps = conn.prepareStatement("update stu set age=? where name=?");
ps.setInt(1, 88);
ps.setString(2, "王五");
ps.executeUpdate();
// 删除记录
ps = conn.prepareStatement("delete from stu where name=?");
ps.setString(1, "李四");
ps.executeUpdate();
// 查询记录
List<Stu> list = new ArrayList<>();
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("select * from stu");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
list.add(new Stu(id, name, age));
}
// 输出结果
for (Stu stu : list) {
System.out.println(stu.getId() + " " + stu.getName() + " " + stu.getAge());
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接
try {
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public List<Stu> findAll() {
List<Stu> list = new ArrayList<>();
// JDBC连接信息
String driver = "com.mysql.jdbc.Driver";
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "root";
Connection conn = null;
try {
// 加载JDBC驱动
Class.forName(driver);
// 建立连接
conn = DriverManager.getConnection(url, username, password);
// 查询记录
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("select * from stu");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
list.add(new Stu(id, name, age));
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接
try {
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
return list;
}
}
```
其中,JDBC连接信息需要根据您自己的数据库配置进行修改。同时,由于添加、修改、删除等操作可能会对数据库造成不可逆的影响,请务必谨慎操作。
相关推荐
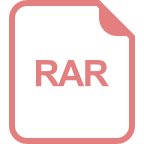
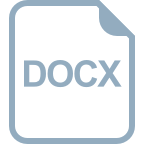
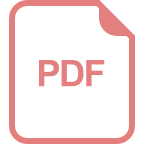













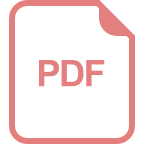