编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果。
时间: 2023-07-10 18:08:50 浏览: 103
可以使用Python中的isupper()、islower()、isdigit()等函数来判断字符的类型,然后进行统计。以下是实现代码:
```python
def count_chars(s):
d = {'upper': 0, 'lower': 0, 'digit': 0, 'other': 0}
for c in s:
if c.isupper():
d['upper'] += 1
elif c.islower():
d['lower'] += 1
elif c.isdigit():
d['digit'] += 1
else:
d['other'] += 1
return d
```
使用示例:
```python
s = 'Hello World! 123'
print(count_chars(s)) # {'upper': 2, 'lower': 8, 'digit': 3, 'other': 3}
```
以上代码中,我们使用一个字典来保存统计结果,并在遍历字符串中的每一个字符时,根据其类型进行相应的统计。最后返回字典即可。
相关问题
Python编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果。
可以使用Python内置的字符串方法isupper、islower、isdigit来判断字符的类别,然后使用字典来存储统计结果。以下是实现代码:
```python
def count_chars(s):
result = {'upper': 0, 'lower': 0, 'digit': 0, 'other': 0}
for char in s:
if char.isupper():
result['upper'] += 1
elif char.islower():
result['lower'] += 1
elif char.isdigit():
result['digit'] += 1
else:
result['other'] += 1
return result
```
其中,s为输入的字符串,result为统计结果的字典。在循环中,每遍历一个字符,就根据其类别更新对应的统计结果。最后返回结果字典即可。
python写代码 编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果
def count_chars(s):
"""
统计字符串中的大写字母、小写字母、数字、其他字符个数
:param s: 字符串
:return: 字典,包含各类字符个数
"""
count_dict = {'upper': 0, 'lower': 0, 'digit': 0, 'other': 0}
for char in s:
if char.isupper():
count_dict['upper'] += 1
elif char.islower():
count_dict['lower'] += 1
elif char.isdigit():
count_dict['digit'] += 1
else:
count_dict['other'] += 1
return count_dict
阅读全文
相关推荐
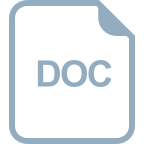
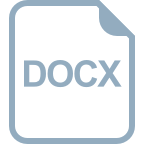
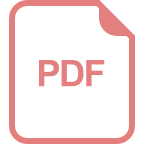












