自己搭建VGG16模型对莺尾花进行识别python
时间: 2023-08-08 10:04:16 浏览: 51
好的,我们可以使用Python中的Keras库来搭建VGG16模型对莺尾花进行识别。下面是实现的步骤:
1. 准备数据集
首先,我们需要准备莺尾花数据集。可以使用sklearn库中的datasets模块获取数据集:
```python
from sklearn.datasets import load_iris
iris = load_iris()
X, y = iris.data, iris.target
```
2. 数据预处理
由于VGG16模型输入的图片尺寸为224x224,而莺尾花数据集中图片的尺寸为4x4,因此我们需要将数据集中的图片进行resize。同时,我们需要进行数据集的划分,将数据集分为训练集和测试集:
```python
from sklearn.model_selection import train_test_split
from tensorflow.keras.utils import to_categorical
from skimage.transform import resize
X = np.array([resize(image, (224, 224)) for image in X])
train_X, test_X, train_y, test_y = train_test_split(X, y, test_size=0.2, random_state=42)
train_y = to_categorical(train_y, num_classes=3)
test_y = to_categorical(test_y, num_classes=3)
```
3. 搭建模型
使用Keras库搭建VGG16模型:
```python
from tensorflow.keras.applications.vgg16 import VGG16
from tensorflow.keras.layers import Flatten, Dense
from tensorflow.keras.models import Model
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
x = base_model.output
x = Flatten()(x)
x = Dense(256, activation='relu')(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(3, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
```
4. 编译模型
编译模型并设置优化器、损失函数和评价指标:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
5. 训练模型
使用训练集训练模型:
```python
model.fit(train_X, train_y, epochs=10, validation_data=(test_X, test_y))
```
6. 评估模型
使用测试集评估模型:
```python
model.evaluate(test_X, test_y)
```
完整代码如下:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from tensorflow.keras.utils import to_categorical
from skimage.transform import resize
from tensorflow.keras.applications.vgg16 import VGG16
from tensorflow.keras.layers import Flatten, Dense
from tensorflow.keras.models import Model
iris = load_iris()
X, y = iris.data, iris.target
X = np.array([resize(image, (224, 224)) for image in X])
train_X, test_X, train_y, test_y = train_test_split(X, y, test_size=0.2, random_state=42)
train_y = to_categorical(train_y, num_classes=3)
test_y = to_categorical(test_y, num_classes=3)
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
x = base_model.output
x = Flatten()(x)
x = Dense(256, activation='relu')(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(3, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(train_X, train_y, epochs=10, validation_data=(test_X, test_y))
model.evaluate(test_X, test_y)
```
相关推荐
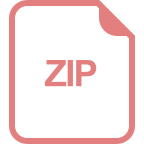
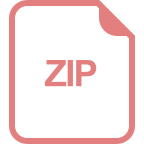














