self.sim_svd = [] print("Calculating the sim_svd...") tbar = tqdm(total=len(self.word_vector)) for word in self.word_vector: if word[0] in self.vocab and word[1] in self.vocab: idx0 = self.vocab.index(word[0]) idx1 = self.vocab.index(word[1]) vector0 = self.result[idx0] vector1 = self.result[idx1] norm0 = np.linalg.norm(vector0) norm1 = np.linalg.norm(vector1) frac0 = np.dot(vector0, vector1) frac1 = norm0 * norm1 if frac1 != 0: sim = np.around(frac0 / frac1, 1) else: sim = 0 self.sim_svd.append(sim) else: self.sim_svd.append(0) tbar.update(1) tbar.close() # print(self.sim_svd) f = open('sim_svd', 'wb') pickle.dump(self.sim_svd, f) f.close()
时间: 2024-02-10 11:35:14 浏览: 121
这段代码看起来是在计算两个词语在词向量空间中的相似度,并将结果保存到了一个文件中。具体来说,代码首先创建了一个空的列表 self.sim_svd,然后通过遍历词向量列表 self.word_vector,计算每个词语与其他词语的相似度,并将结果添加到 self.sim_svd 中。计算相似度的公式使用的是余弦相似度,即两个向量的点积除以它们的模长之积。如果两个词语都在词汇表 self.vocab 中,就可以通过它们在词汇表中的索引找到它们在词向量矩阵 self.result 中对应的向量,并计算它们的点积和模长,从而得到它们的相似度;如果两个词语中有任何一个不在词汇表中,则将它们的相似度设为 0。最后,代码将计算得到的相似度列表 self.sim_svd 保存到一个名为 'sim_svd' 的二进制文件中,使用 pickle.dump() 函数实现。
相关问题
def SVG_process(self): # SVG方法 self.get_subword_vector() M = np.zeros((len(self.vocab), len(self.vocab))) df = pd.DataFrame(M, index=self.vocab, columns=self.vocab) print("Calculating the subword vector...") # 利用dataframe的字符串索引功能,使用子词向量进行计数,记录子词向量在词表中的出现频率 tbar = tqdm(total=len(self.subword_vector)) for i in self.subword_vector: try: df.at[i[0], i[1]] += 1 except: pass tbar.update(1) tbar.close() M = np.array(df) print(np.max(M)) svd = TruncatedSVD(n_components=3) self.result = svd.fit_transform(M) print(self.result.shape) def SGNS_process(self): print("Calculating the sim_sgns...") logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s') self.sim_sgns = [] vec_sgns = Word2Vec(LineSentence('dataset.txt'), vector_size=100, window=2, sg=1, hs=0, min_count=1, workers=multiprocessing.cpu_count()) tbar = tqdm(total=len(self.word_vector)) for word in self.word_vector: try: self.sim_sgns.append(vec_sgns.wv.similarity(word[0], word[1])) except: self.sim_sgns.append(0) tbar.update(1) tbar.close() # print(self.sim_sgns) f = open('sim_sgns', 'wb') pickle.dump(self.sim_sgns, f) f.close()
这段代码中包含了两个方法,一个是SVG_process,另一个是SGNS_process。
SVG_process方法中,首先调用了get_subword_vector方法获取词表中的子词向量,然后使用一个二维数组M初始化一个DataFrame对象df,用于记录子词向量在词表中的出现频率。接着遍历子词向量列表,利用DataFrame的字符串索引功能将子词向量的出现次数记录在df中。最后使用TruncatedSVD对M进行奇异值分解,得到降维后的结果self.result。
SGNS_process方法中,首先使用Word2Vec读取文本数据集,构建词向量模型vec_sgns。然后遍历词向量列表,使用wv.similarity计算每个词向量之间的相似度,并将结果保存在self.sim_sgns列表中。最后将self.sim_sgns保存到文件中。
def output(self): f = open('2020522085.txt', 'w' ,encoding='utf-8') with open('sim_svd', 'rb') as f1: self.sim_svd = pickle.load(f1) f1.close() with open('sim_sgns', 'rb', encoding='utf-8') as f1: self.sim_sgns = pickle.load(f1) f1.close() for i in range(500): f.write(str(' '.join(self.word_vector[i])) + ' ' + str(self.sim_svd[i]) + ' ' + str(self.sim_sgns[i]) + '\n') f.close()
这段代码看起来是在将之前计算得到的两种相似度(self.sim_svd 和 self.sim_sgns)以及对应的词语对(self.word_vector)输出到一个名为 '2020522085.txt' 的文本文件中。
具体来说,代码首先创建了一个文件对象 f,指定其文件名为 '2020522085.txt',打开方式为写入('w'),并指定文件编码为 'utf-8'。接着,代码使用 pickle.load() 函数从之前保存的二进制文件 'sim_svd' 和 'sim_sgns' 中分别读取相似度列表 self.sim_svd 和 self.sim_sgns,注意需要使用二进制模式打开文件。然后,代码使用 for 循环遍历前 500 个词语对,将它们的词语和相似度信息以一定的格式写入文件 f 中。具体来说,对于每个词语对,代码将其两个词语使用空格连接起来,然后接上两个相似度值,中间使用若干个空格分隔,最后加上一个换行符。文件写入完成后,代码使用 f.close() 关闭文件对象。
阅读全文
相关推荐
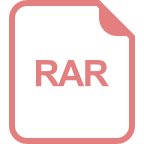
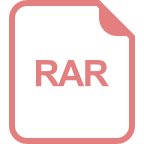
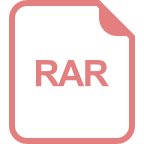
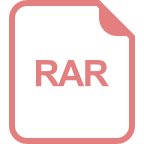
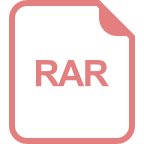
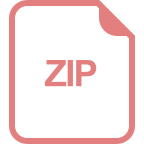
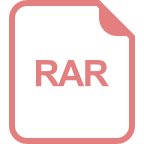
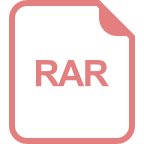
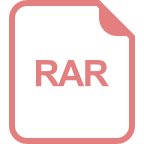
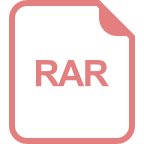
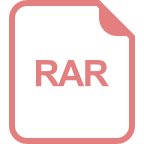
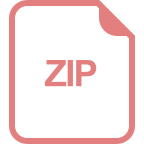
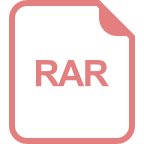
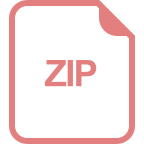