实现俄罗斯方块的python代码
时间: 2023-04-08 19:01:42 浏览: 132
我可以回答这个问题。以下是一个简单的实现俄罗斯方块的Python代码:
```python
import random
# 定义方块的形状
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
# 定义方块的颜色
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255)
]
# 定义游戏区域的大小
board_width = 10
board_height = 20
# 定义游戏区域
board = [[0] * board_width for _ in range(board_height)]
# 定义方块的初始位置
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = board_width // 2 - len(current_shape[0]) // 2
current_y = 0
# 定义游戏循环
while True:
# 绘制游戏区域
for y in range(board_height):
for x in range(board_width):
if board[y][x] == 0:
pygame.draw.rect(screen, (128, 128, 128), (x * 20, y * 20, 20, 20))
else:
pygame.draw.rect(screen, colors[board[y][x]], (x * 20, y * 20, 20, 20))
# 绘制当前方块
for y in range(len(current_shape)):
for x in range(len(current_shape[0])):
if current_shape[y][x] != 0:
pygame.draw.rect(screen, colors[current_color], ((current_x + x) * 20, (current_y + y) * 20, 20, 20))
# 更新屏幕
pygame.display.update()
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# 移动方块
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
if current_x > 0:
current_x -= 1
if keys[pygame.K_RIGHT]:
if current_x < board_width - len(current_shape[0]):
current_x += 1
if keys[pygame.K_DOWN]:
if current_y < board_height - len(current_shape):
current_y += 1
# 判断方块是否落地
if current_y + len(current_shape) == board_height or \
any(board[current_y + y][current_x + x] != 0 for y in range(len(current_shape)) for x in range(len(current_shape[0]))):
# 将方块放到游戏区域中
for y in range(len(current_shape)):
for x in range(len(current_shape[0])):
if current_shape[y][x] != 0:
board[current_y + y][current_x + x] = current_color
# 生成新的方块
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors) - 1)
current_x = board_width // 2 - len(current_shape[0]) // 2
current_y = 0
# 消除满行
for y in range(board_height):
if all(board[y][x] != 0 for x in range(board_width)):
for y2 in range(y, 0, -1):
board[y2] = board[y2 - 1].copy()
board[0] = [0] * board_width
# 延迟一段时间
pygame.time.delay(100)
```
这段代码使用 Pygame 库实现了一个简单的俄罗斯方块游戏。
阅读全文
相关推荐
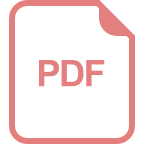


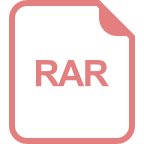
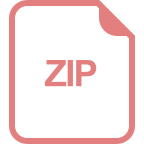
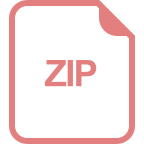
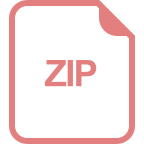
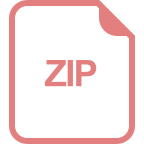
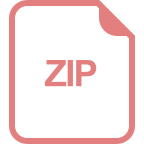
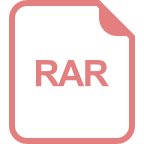
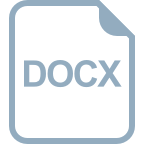