c#如何获取路径有几种方法
时间: 2024-02-17 22:01:29 浏览: 131
是的,C# 中获取路径有多种方法,具体取决于您需要获取的路径类型。以下是一些常见的获取路径的方法:
1. 获取应用程序的执行路径:
```csharp
string appPath = AppDomain.CurrentDomain.BaseDirectory;
```
2. 获取特定文件的路径:
```csharp
string filePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "filename.txt");
```
3. 获取当前工作目录的路径:
```csharp
string currentDirectoryPath = Directory.GetCurrentDirectory();
```
4. 获取特定目录的路径:
```csharp
string directoryPath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.Desktop), "myDirectory");
```
5. 获取程序集的路径:
```csharp
string assemblyPath = Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location);
```
这些方法可以根据您的需要进行调整和组合。希望这可以帮助您!
相关问题
c#通过路径获取图片文件名
在C#中,你可以使用`System.IO`命名空间下的`Directory.GetFiles()`或者`File.ReadAllLines()`方法配合正则表达式来从路径中获取图片文件名。这里是一个基本的例子:
```csharp
using System;
using System.IO;
using System.Text.RegularExpressions;
public string[] GetImageFiles(string path)
{
// 获取指定目录下的所有文件
string[] files = Directory.GetFiles(path, "*.jpg;*.png;*.gif"); // 假设我们只关心这几种常见的图片格式
if (files.Length > 0)
{
// 使用正则表达式匹配文件名(忽略路径)
var regex = new Regex(@"^(?:(?:[a-z]:)?\\|/)((?:\w|\.\-)+\.)(?:jpg|png|gif)$");
return files.Select(file => regex.Match(file).Groups[1].Value).ToArray();
}
else
{
return null; // 如果没有找到文件,返回null
}
}
// 调用函数并打印结果
string[] imageNames = GetImageFiles(@"C:\YourDirectoryPath");
if (imageNames != null)
{
foreach (string fileName in imageNames)
{
Console.WriteLine(fileName);
}
}
else
{
Console.WriteLine("No image files found.");
}
C#获取图片的绝对路径
在C#中,获取图片的绝对路径通常是指获取存储在文件系统中的图片文件的完整路径。以下是获取图片绝对路径的几种常见方法:
1. 使用`System.IO`命名空间下的`FileInfo`类来获取。例如,如果你已经有一个文件路径或者从某个源得到了一个图片文件名,你可以这样做:
```csharp
FileInfo fileInfo = new FileInfo("图片文件名");
string absolutePath = fileInfo.FullName;
```
2. 如果图片是在应用程序的资源中,可以通过资源管理器获取其绝对路径。首先需要确定资源的类型和位置,然后使用相应的API获取资源路径。
```csharp
// 假设图片是嵌入在程序集中的资源
string resourceName = "命名空间.图片资源名";
Assembly assembly = Assembly.GetExecutingAssembly();
string resourcePath = assembly.GetManifestResourceNames().Single(str => str.EndsWith(resourceName));
string absolutePath = assembly.GetManifestResourceStream(resourcePath).ToString();
```
3. 在Web应用中,如果你需要获取上传图片的绝对路径,可以在上传图片后使用服务器的文件系统来指定路径:
```csharp
string fileName = Path.GetFileName上传时的文件名);
string folderPath = Server.MapPath("~/上传文件夹路径");
string filePath = Path.Combine(folderPath, fileName);
string absolutePath = Path.GetFullPath(filePath);
```
请注意,Web应用中的路径会依赖于服务器的配置和应用程序的目录结构。
阅读全文
相关推荐






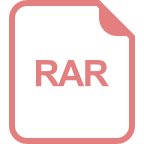




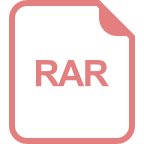
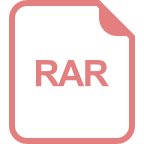
