Part part=request.getPart("companyPic"); String fileName=part.getSubmittedFileName(); String newFileName=System.currentTimeMillis()+fileName.substring(fileName.lastIndexOf(".")); String filepath =getServletContext().getRealPath("/"); filepath=filepath.substring(0,filepath.indexOf(getServletContext().getServletContextName())); filepath=filepath+"upload/companyPic"; getServletContext().log("上传路径为:"+filepath) ; File f=new File(filepath); if(!f.exists()) f.mkdirs(); part.write(filepath+"/"+newFileName);如何更改上传地址
时间: 2023-06-08 16:06:04 浏览: 61
要更改上传地址,需要更改以下代码中的"upload/companyPic"部分:
filepath = getServletContext().getRealPath("/");
filepath = filepath.substring(0, filepath.indexOf(getServletContext().getServletContextName()));
filepath = filepath + "upload/companyPic";
您可以将“upload/companyPic”更改为新的上传路径,然后保存并重新运行代码即可。请确保新路径存在并具有正确的权限。
相关问题
用form表单做文件上传时候,在servlet页面使用Part filePart = request.getPart("file1");获取该文件,获取的值为null原因是前面传过来的路径是受保护的,如何处理
当使用form表单上传文件时,需要确保在servlet中使用正确的multipart配置。在servlet中,可以将multipart配置设置为@MultipartConfig注解或在web.xml中进行配置。如果没有正确配置multipart,可能会导致无法获取上传的文件。
另外,如果获取的Part对象为null,可能是因为没有在form表单中正确指定文件上传的input标签的name属性。确保name属性与servlet中获取Part对象的名称相匹配。
如果上传路径受保护,可以考虑将上传文件保存到临时目录中,或者将上传文件保存到另一个目录中,该目录不受保护。在servlet中,可以通过调用Part对象的write()方法将上传文件保存到指定的路径中。
以下是一个示例代码片段,用于演示如何正确处理文件上传:
```java
@MultipartConfig(fileSizeThreshold = 1024 * 1024 * 2, // 2MB
maxFileSize = 1024 * 1024 * 10, // 10MB
maxRequestSize = 1024 * 1024 * 50) // 50MB
public class FileUploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取上传的文件
Part filePart = request.getPart("file1");
if (filePart != null) {
// 保存文件到指定路径中
String fileName = filePart.getSubmittedFileName();
filePart.write("path/to/save/" + fileName);
// 做其他处理
}
}
}
```
在上面的示例中,@MultipartConfig注解指定了上传文件的大小限制和临时文件存储路径。在servlet中,通过调用request.getPart()方法获取上传的文件,然后将其保存到指定路径中。
request.getPart
request.getPart() is a method in the Servlet API that is used to retrieve a specific part of a multipart request. A multipart request is a type of HTTP request that can contain multiple parts, such as files or form data.
The getPart() method takes a String parameter that represents the name of the part to retrieve. It returns an instance of the Part interface, which represents a single part of the multipart request.
Here is an example usage of the getPart() method:
```
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Part filePart = request.getPart("file");
// do something with the filePart
}
```
In this example, the getPart() method is used to retrieve the part of the request with the name "file". The returned Part object can then be used to access the content of the file that was uploaded as part of the request.
相关推荐
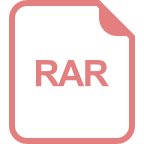
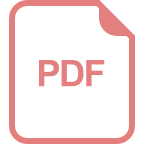
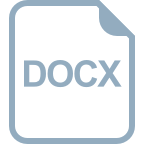












