编写一个函数,接受一个字符串作为输入,并返回该字符串中所有大写字母的个数。
时间: 2024-04-17 07:24:18 浏览: 220
好的,以下是一个编写函数来统计字符串中写字母个数的例子:
```python
def count_uppercase_letters(input_string):
count = 0
for char in input_string:
if char.isupper():
count += 1
return count
# 测试例子
string = "Hello, World!"
uppercase_count = count_uppercase_letters(string)
print(uppercase_count)
```
输出结果:
```
2
```
该函数使用了一个循环遍历字符串中的每个字符,使用 `isupper()` 方法来检查字符是否为大写字母。如果是大写字母,则计数器 `count` 值加一。最后返回计数器的值作为结果。
希望这个例子能够帮助您理解如何编写一个函数来统计字符串中大写字母的个数。如果您有任何疑问,请随时告诉我。
相关问题
编写一个函数接收一个字符串,返回输入字符串中的大写字母、小写字母和 数字的个数。
```
def count_chars(string):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
return (upper_count, lower_count, digit_count, other_count)
```
python编写一个函数接收一个字符串, 返回输入字符串中的大写字母、小写字母和 数字的个数
可以使用Python内置函数isupper()、islower()、isdigit()来判断字符的类型,然后统计个数即可。具体实现如下:
def count_chars(s):
upper_count =
lower_count =
digit_count =
other_count =
for c in s:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
return (upper_count, lower_count, digit_count, other_count)
# 测试
s = "Hello, World! 123"
print(count_chars(s)) # 输出:(2, 8, 3, 4)
阅读全文
相关推荐
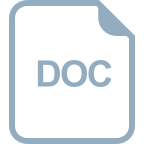
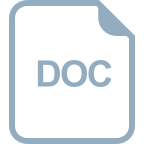
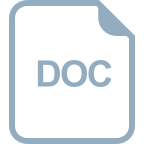













