c#模拟键盘输入
时间: 2023-07-10 17:11:36 浏览: 114
在 C# 中模拟键盘输入可以使用 SendKeys 类。下面是一个简单的示例:
```csharp
using System.Windows.Forms;
// 模拟键盘输入
SendKeys.Send("Hello World!");
```
上面的代码将会模拟键盘输入字符串 "Hello World!"。你也可以模拟键盘上的特殊按键,例如回车键、Tab 键等等。例如:
```csharp
using System.Windows.Forms;
// 模拟按下回车键
SendKeys.Send("{ENTER}");
// 模拟按下 Tab 键
SendKeys.Send("{TAB}");
```
注意:在模拟键盘输入时,你需要确保当前窗口是活动窗口,否则模拟键盘输入可能会失败。
相关问题
c#模拟键盘输入操作
在 C# 中,你可以使用 `SendKeys` 类来自动生成键盘输入,模拟用户在应用程序中敲击按键的行为。`SendKeys` 提供了一组静态方法,可以发送单个键、组合键、字符串以及特殊的控制字符。以下是一个简单的例子:
```csharp
using System.Windows.Forms;
// 模拟按下 'A' 键
SendKeys.Send("A");
// 模拟 Shift + A
SendKeys.Send("{shift}{a}");
// 模拟输入文本 "Hello World"
SendKeys.SendWait("Hello World");
// 等待用户按下回车键
SendKeys.SendWait(Keys.Enter);
```
注意:
1. `Send` 和 `SendWait` 的区别在于,`Send` 立即返回并继续执行下一行代码,而 `SendWait` 会阻塞直到输入的操作完成。
2. 使用这种方法时要谨慎,因为它可能会干扰到其他程序的正常运行,特别是在处理窗口焦点或敏感事件时。
c#模拟键盘输入到指定位置
如果你想将模拟键盘输入发送到指定的位置,可以使用Windows API函数`SetForegroundWindow`和`SendInput`。以下是一个示例代码,它将模拟按下"Hello World"键,并将其发送到指定的文本框中:
```csharp
using System;
using System.Diagnostics;
using System.Runtime.InteropServices;
// 设置前台窗口并模拟键盘输入
[DllImport("user32.dll")]
private static extern bool SetForegroundWindow(IntPtr hWnd);
[DllImport("user32.dll")]
private static extern uint SendInput(uint nInputs, INPUT[] pInputs, int cbSize);
private const int INPUT_KEYBOARD = 1;
private const uint KEYEVENTF_EXTENDEDKEY = 0x0001;
private const uint KEYEVENTF_KEYUP = 0x0002;
[StructLayout(LayoutKind.Sequential)]
private struct INPUT
{
public int type;
public INPUTUNION union;
}
[StructLayout(LayoutKind.Explicit)]
private struct INPUTUNION
{
[FieldOffset(0)]
public KEYBDINPUT keyboardInput;
}
[StructLayout(LayoutKind.Sequential)]
private struct KEYBDINPUT
{
public ushort wVk;
public ushort wScan;
public uint dwFlags;
public uint time;
public IntPtr dwExtraInfo;
}
// 获取窗口句柄
Process process = Process.GetProcessesByName("YourProcessName")[0];
IntPtr hwnd = process.MainWindowHandle;
// 设置前台窗口
SetForegroundWindow(hwnd);
// 模拟键盘输入
string text = "Hello World";
foreach (char c in text)
{
INPUT[] inputs = new INPUT[2];
inputs[0].type = INPUT_KEYBOARD;
inputs[0].union.keyboardInput.wVk = 0;
inputs[0].union.keyboardInput.wScan = (ushort)c;
inputs[0].union.keyboardInput.dwFlags = KEYEVENTF_UNICODE;
inputs[0].union.keyboardInput.time = 0;
inputs[0].union.keyboardInput.dwExtraInfo = IntPtr.Zero;
inputs[1].type = INPUT_KEYBOARD;
inputs[1].union.keyboardInput.wVk = 0;
inputs[1].union.keyboardInput.wScan = (ushort)c;
inputs[1].union.keyboardInput.dwFlags = KEYEVENTF_UNICODE | KEYEVENTF_KEYUP;
inputs[1].union.keyboardInput.time = 0;
inputs[1].union.keyboardInput.dwExtraInfo = IntPtr.Zero;
SendInput(2, inputs, Marshal.SizeOf(typeof(INPUT)));
}
```
在代码中,我们首先使用`GetProcessesByName`函数获取指定进程的句柄,然后使用`SetForegroundWindow`函数将其设置为前台窗口。接下来,我们使用`SendInput`函数模拟键盘输入,将每个字符一个接一个地发送到指定的文本框中。你可以根据需要修改代码以适应你的应用程序。
阅读全文
相关推荐
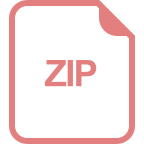
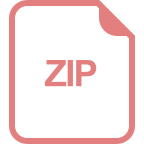
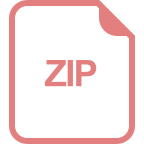
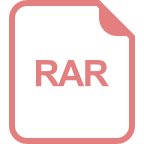
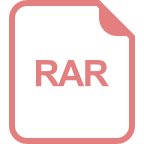
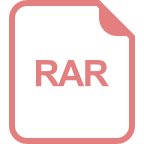
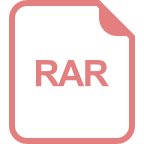
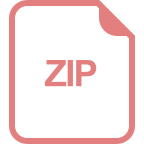

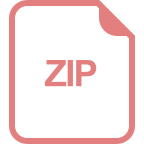
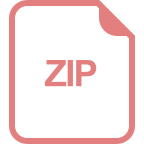