写一个vuex的购物车案例
时间: 2023-08-19 22:03:17 浏览: 56
好的,下面是一个简单的Vuex购物车案例:
1. 在Vue.js应用程序中安装Vuex库。
```
npm install vuex --save
```
2. 在Vuex中定义状态(state),包括购物车中的商品数组、总金额和总数量。
```
const store = new Vuex.Store({
state: {
cartItems: [],
cartTotal: 0,
cartQuantity: 0
},
mutations: {
addToCart(state, item) {
// 检查商品是否已存在于购物车中
const cartItem = state.cartItems.find(i => i.id === item.id)
if (cartItem) {
cartItem.quantity++
} else {
state.cartItems.push({
id: item.id,
name: item.name,
price: item.price,
quantity: 1
})
}
// 更新购物车总金额和总数量
state.cartTotal += item.price
state.cartQuantity++
},
removeFromCart(state, item) {
// 从购物车中删除商品
const cartItem = state.cartItems.find(i => i.id === item.id)
if (cartItem) {
if (cartItem.quantity > 1) {
cartItem.quantity--
} else {
state.cartItems = state.cartItems.filter(i => i.id !== item.id)
}
// 更新购物车总金额和总数量
state.cartTotal -= item.price
state.cartQuantity--
}
}
}
})
```
3. 在Vue.js组件中使用Vuex状态。
```
<template>
<div>
<ul>
<li v-for="item in cartItems" :key="item.id">
{{ item.name }} - {{ item.price }} x {{ item.quantity }}
<button @click="removeFromCart(item)">Remove</button>
</li>
</ul>
<p>Total: {{ cartTotal }}</p>
<p>Quantity: {{ cartQuantity }}</p>
<button @click="addToCart({ id: 1, name: 'Product A', price: 10 })">Add to Cart</button>
</div>
</template>
<script>
import { mapState, mapMutations } from 'vuex'
export default {
computed: mapState([
'cartItems',
'cartTotal',
'cartQuantity'
]),
methods: mapMutations([
'addToCart',
'removeFromCart'
])
}
</script>
```
在这个组件中,我们使用mapState辅助函数从Vuex中获取购物车中的商品、总金额和总数量,并使用mapMutations辅助函数调用addToCart和removeFromCart mutations来更改购物车中的商品。我们还添加了一个按钮来模拟将商品添加到购物车中。
相关推荐
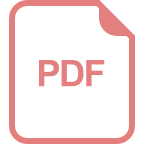
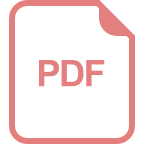











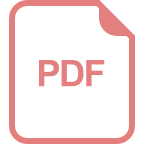
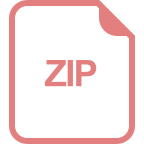