在Java中,对比两个不同实体类的list属性值是否一样,代码实现的所有方法
时间: 2023-12-12 07:02:01 浏览: 42
有多种实现方法,以下是其中几种比较常见的方法:
1. 重写equals方法:在实体类中重写equals方法,在该方法中判断属性值是否相等,然后在比较两个list时,逐个比较每个元素的equals方法返回值是否为true,如果全部相等,则两个list相等。示例代码如下:
```java
public class Entity {
private int id;
private String name;
// getter和setter方法省略
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Entity)) {
return false;
}
Entity entity = (Entity) obj;
return id == entity.id && Objects.equals(name, entity.name);
}
}
```
```java
public boolean compareList(List<Entity> list1, List<Entity> list2) {
if (list1 == list2) {
return true;
}
if (list1 == null || list2 == null || list1.size() != list2.size()) {
return false;
}
for (int i = 0; i < list1.size(); i++) {
if (!list1.get(i).equals(list2.get(i))) {
return false;
}
}
return true;
}
```
2. 使用Set:将两个list转换为Set,然后比较两个Set是否相等。示例代码如下:
```java
public boolean compareList(List<Entity> list1, List<Entity> list2) {
if (list1 == list2) {
return true;
}
if (list1 == null || list2 == null || list1.size() != list2.size()) {
return false;
}
Set<Entity> set1 = new HashSet<>(list1);
Set<Entity> set2 = new HashSet<>(list2);
return set1.equals(set2);
}
```
3. 使用Stream API:使用Stream API将两个list转换为Stream,然后使用allMatch方法比较两个Stream中每个元素是否相等。示例代码如下:
```java
public boolean compareList(List<Entity> list1, List<Entity> list2) {
if (list1 == list2) {
return true;
}
if (list1 == null || list2 == null || list1.size() != list2.size()) {
return false;
}
return IntStream.range(0, list1.size())
.allMatch(i -> list1.get(i).equals(list2.get(i)));
}
```
4. 使用Apache Commons Collections库:使用org.apache.commons.collections4.CollectionUtils.isEqualCollection方法比较两个list是否相等。示例代码如下:
```java
public boolean compareList(List<Entity> list1, List<Entity> list2) {
if (list1 == list2) {
return true;
}
if (list1 == null || list2 == null || list1.size() != list2.size()) {
return false;
}
return CollectionUtils.isEqualCollection(list1, list2);
}
```
相关推荐
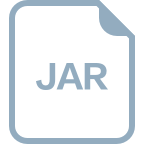
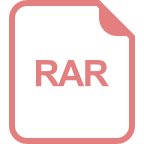














