IAR中的255mn-l01与主芯片通过串口连接,通过主芯片发送数据给该器件而该器件返回一个应答信号时代表两者之间的串口通信是正常的,如何通过代码实现这一现象?代码详解
时间: 2024-09-09 10:10:33 浏览: 41
IAR集成开发环境主要用于基于ARM Cortex-M系列微控制器的项目开发,如果要在C语言中实现这种串口通信,通常我们会使用标准的UART(Universal Asynchronous Receiver/Transmitter)功能。这里我会提供一个基本的示例,假设主芯片使用的是Keil uVision的库函数:
```c
#include "iar.h" // 包含包含IAR特定头文件
#include "stdio.h"
#include "uart.h" // 如果有专门的UART库
// 定义串口配置
const UART_CONFIG uart_config = {
.baud_rate = 9600, // 设置波特率
.data_bits = UART_BITS_8, // 数据位数
.stop_bits = UART_STOPBITS_1, // 停止位
.parity = UART_NO_PARITY, // 没有校验位
};
void send_data_to_device(const char *data) {
// 打开串口
if (uart_open(UART_PORT, &uart_config)) {
printf("Failed to open UART.\n");
return;
}
// 发送数据
size_t bytes_sent = uart_write(UART_PORT, data, strlen(data));
if (bytes_sent == 0) {
printf("Failed to send data.\n");
} else {
printf("Sent %zd bytes of data.\n", bytes_sent);
}
// 关闭串口
uart_close(UART_PORT);
}
void receive_and_check_response() {
char buffer[16]; // 接收数据的缓冲区
size_t bytes_received = uart_read(UART_PORT, buffer, sizeof(buffer));
if (bytes_received > 0) {
printf("Received response: %s\n", buffer); // 输出接收到的数据
// 根据实际需要检查响应是否符合预期
if (/* 检查响应 */ true) {
printf("Response is valid.\n");
} else {
printf("Invalid response.\n");
}
} else {
printf("No response received.\n");
}
}
int main(void) {
// 初始化设备并设置为监听模式
initialize_device();
// 主循环,发送数据并接收应答
while (true) {
send_data_to_device("Hello from main!");
receive_and_check_response();
// 等待一段时间再继续下一轮循环
delay_ms(1000);
}
return 0;
}
```
这个示例展示了如何在一个无限循环中发送数据到外部设备,并检查它是否返回了预期的响应。你需要根据实际硬件和协议调整`send_data_to_device`、`receive_and_check_response`以及相关的初始化函数。
阅读全文
相关推荐
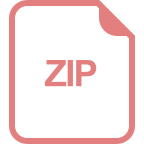
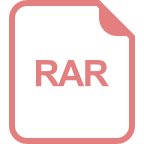
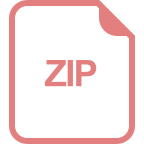















