pandas查找inf值
时间: 2023-06-29 09:20:41 浏览: 58
你可以使用 Pandas 中的 `isinf()` 函数来查找 `inf` 值。
以下是一个示例代码:
```python
import pandas as pd
import numpy as np
# 创建一个包含 inf 值的 DataFrame
df = pd.DataFrame({'A': [1, 2, np.inf, 4],
'B': [np.inf, 6, 7, 8]})
# 查找 DataFrame 中的 inf 值
df_inf = df[df.isin([np.inf, -np.inf]).any(1)]
print(df_inf)
```
输出结果如下:
```
A B
2 inf inf
```
在上面的代码中,我们首先创建了一个包含 `inf` 值的 DataFrame,然后使用 `isin()` 函数和 `any()` 函数来查找包含 `inf` 值的行,并将其保存到一个新的 DataFrame `df_inf` 中。
相关问题
pandas查找缺失值
在Pandas中,缺失值有三种:np.nan (Not a Number)、None和pd.NaT(时间格式的空值)。可以使用isnull()方法来查找缺失值,该方法返回True表示此处为缺失值。可以对数据集进行切片来找到缺失值。另外,可以使用fillna()方法来填充缺失值,该方法可以使用不同的填充方式,例如使用平均值、中位数或前一个/后一个非缺失值来填充。如果缺失值的数量很少,也可以考虑直接删除缺失值所在的行或列。
pandas -inf
在Pandas中,-inf表示负无穷大。可以使用df.replace([np.inf, -np.inf], np.nan)将数据框中的正负无穷替换为NaN,然后使用dropna()方法删除含有NaN的行或列。具体可以使用df.replace([np.inf, -np.inf], np.nan).dropna()删除含有正负无穷的行或列。另外,df.dropna(how='all')可以删除所有值为缺失值的行,df.dropna(axis=1)可以删除含有缺失值的列,df.dropna(axis=1, how='all')可以删除所有列中所有值均为缺失值的列。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Pandas缺失值inf与nan处理实践](https://blog.csdn.net/xiaoyw/article/details/126241356)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
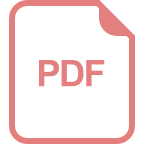
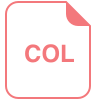
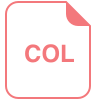
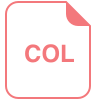









