js html格式转译成tsx格式
时间: 2023-07-19 15:08:37 浏览: 292
将 HTML 格式转换成 TSX(TypeScript JSX)格式需要以下步骤:
1. 将 HTML 格式的代码复制到一个字符串变量中。
2. 使用正则表达式或者 DOM 解析器将字符串解析成 DOM 对象。
3. 遍历 DOM 对象,将每个节点转换成相应的 TSX 代码。
4. 将所有转换后的 TSX 代码拼接成一个字符串,并返回该字符串。
以下是一个示例代码,用于将 HTML 格式的代码转换成 TSX 格式:
```javascript
function convertHtmlToTsx(html) {
// 使用正则表达式将字符串解析成 DOM 对象
const domParser = new DOMParser();
const doc = domParser.parseFromString(html, 'text/html');
// 遍历 DOM 对象,将每个节点转换成相应的 TSX 代码
const elements = Array.from(doc.body.children);
const tsxElements = elements.map((element) => {
const tagName = element.tagName.toLowerCase();
const attributes = Array.from(element.attributes).map((attribute) => {
return `${attribute.nodeName}="${attribute.nodeValue}"`;
}).join(' ');
const children = Array.from(element.children).map((child) => {
if (child.nodeType === Node.TEXT_NODE) {
// 如果是文本节点,直接返回文本内容
return child.textContent.trim();
} else {
// 递归调用 convertHtmlToTsx 函数,转换子元素
return convertHtmlToTsx(child.outerHTML);
}
}).join('\n');
return `<${tagName} ${attributes}>${children}</${tagName}>`;
});
// 将所有转换后的 TSX 代码拼接成一个字符串,并返回该字符串
return tsxElements.join('\n');
}
```
使用示例:
```javascript
const html = `
<div class="container">
<h1>Hello, world!</h1>
<p>This is a paragraph.</p>
</div>
`;
const tsx = convertHtmlToTsx(html);
console.log(tsx);
```
输出结果:
```typescript
<div className="container">
<h1>Hello, world!</h1>
<p>This is a paragraph.</p>
</div>
```
阅读全文
相关推荐
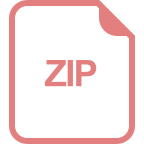
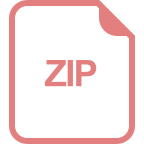
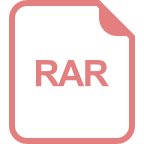
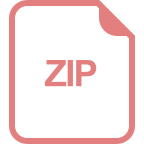
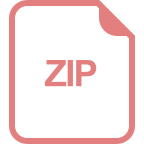