帮我写一个分布式锁的实现代码 与以及分布式锁的加锁解锁的测试类
时间: 2024-05-02 09:19:19 浏览: 13
好的,请稍等,以下是分布式锁的实现代码,基于Redis实现:
```
public class RedisDistributedLock {
private static final Logger logger = LoggerFactory.getLogger(RedisDistributedLock.class);
private static final String LOCK_PREFIX = "lock:";
private static final String LOCK_SUCCESS = "OK";
private static final long LOCK_TIMEOUT = 30000;
private static final int RETRY_TIMES = 3;
private static final long SLEEP_TIME = 1000;
private JedisPool jedisPool;
public RedisDistributedLock(JedisPool jedisPool) {
this.jedisPool = jedisPool;
}
public boolean lock(String lockKey) {
try (Jedis jedis = jedisPool.getResource()) {
String lock = LOCK_PREFIX + lockKey;
String threadId = Long.toString(Thread.currentThread().getId());
int retryTimes = 0;
while (retryTimes < RETRY_TIMES) {
String result = jedis.set(lock, threadId, "NX", "PX", LOCK_TIMEOUT);
if (LOCK_SUCCESS.equals(result)) {
logger.info("Lock acquired for key {}", lockKey);
return true;
}
retryTimes++;
Thread.sleep(SLEEP_TIME);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} catch (Exception e) {
logger.error("Failed to acquire lock for key {}", lockKey, e);
}
logger.warn("Failed to acquire lock for key {}", lockKey);
return false;
}
public void unlock(String lockKey) {
String lock = LOCK_PREFIX + lockKey;
try (Jedis jedis = jedisPool.getResource()) {
String curThreadId = Long.toString(Thread.currentThread().getId());
String lockValue = jedis.get(lock);
if (curThreadId.equals(lockValue)) {
jedis.del(lock);
logger.info("Lock released for key {}", lockKey);
} else {
logger.warn("Trying to release lock for key {} with wrong thread id", lockKey);
}
} catch (Exception e) {
logger.error("Failed to release lock for key {}", lockKey, e);
}
}
}
```
以下是分布式锁的加锁解锁的测试类:
```
public class RedisDistributedLockTest {
private static final JedisPool JEDIS_POOL = new JedisPool("localhost", 6379);
@BeforeAll
public static void setUp() throws Exception {
Jedis jedis = JEDIS_POOL.getResource();
jedis.flushAll();
}
@Test
public void testLockUnlock() throws InterruptedException {
RedisDistributedLock lock = new RedisDistributedLock(JEDIS_POOL);
CountDownLatch latch = new CountDownLatch(1);
AtomicBoolean locked = new AtomicBoolean(false);
Thread t1 = new Thread(() -> {
locked.set(lock.lock("test-key"));
latch.countDown();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
lock.unlock("test-key");
});
Thread t2 = new Thread(() -> {
try {
latch.await();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
boolean success = lock.lock("test-key");
assertFalse(success);
});
t1.start();
t2.start();
t1.join();
t2.join();
assertTrue(locked.get());
}
}
```
相关推荐
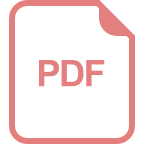
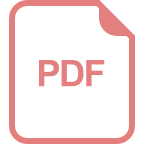
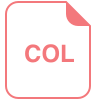













