C# IE浏览器 判断是否已经打开了指定Url
时间: 2023-11-24 22:06:09 浏览: 37
你可以使用 System.Diagnostics.Process 类来检查系统中是否有指定的进程在运行。首先,你需要获取到指定浏览器的进程名称。例如,IE浏览器的进程名称为 iexplore.exe。然后,你可以使用 Process.GetProcessesByName 方法来获取指定名称的所有进程,然后遍历这些进程,检查它们的主窗口句柄是否包含指定的 URL。
以下是一个示例代码,用于检查 IE 浏览器是否已经打开了指定的 URL:
```csharp
using System.Diagnostics;
public static bool IsUrlOpened(string url)
{
string processName = "iexplore"; // IE浏览器的进程名称
Process[] processes = Process.GetProcessesByName(processName);
foreach (Process process in processes)
{
IntPtr handle = process.MainWindowHandle;
if (handle != IntPtr.Zero)
{
string windowUrl = GetUrlFromWindowHandle(handle);
if (windowUrl == url)
{
return true;
}
}
}
return false;
}
private static string GetUrlFromWindowHandle(IntPtr handle)
{
const int maxUrlLength = 2048;
StringBuilder sb = new StringBuilder(maxUrlLength);
int length = NativeMethods.GetWindowText(handle, sb, maxUrlLength);
if (length > 0)
{
string windowTitle = sb.ToString();
if (windowTitle.StartsWith("about:") || windowTitle.StartsWith("http"))
{
return windowTitle;
}
}
return string.Empty;
}
internal static class NativeMethods
{
[DllImport("user32.dll", CharSet = CharSet.Auto)]
internal static extern int GetWindowText(IntPtr hWnd, StringBuilder lpString, int nMaxCount);
}
```
上面的代码中,我们使用了 `GetProcessesByName` 方法来获取所有名为 iexplore 的进程。然后,我们遍历这些进程的主窗口句柄,通过 `GetWindowText` 方法获取窗口标题,从而判断窗口是否包含指定的 URL。
请注意,这种方法有一些限制。首先,它仅适用于 IE 浏览器,如果你想检查其他浏览器是否打开了指定的 URL,你需要修改进程名称。此外,这种方法还可能会误判,因为一个浏览器窗口可以同时包含多个标签页,每个标签页可能包含不同的 URL,我们只能获取窗口的标题,无法精确判断哪个标签页包含指定的 URL。
相关推荐
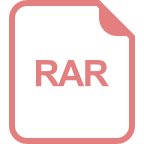
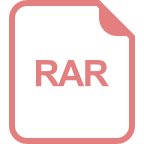
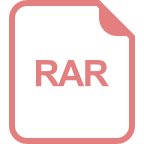
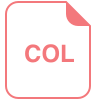













