qlearning代码
时间: 2023-07-24 09:43:08 浏览: 98
Sure, here's an example of Q-learning code in Python:
```python
import numpy as np
# Define the Q-learning
def q_learning(env, num_episodes, learning, discount_factor):
# Initialize Q-table with zeros
q_table =.zeros((env.num_states, env.num_actions # Run Q-learning algorithm
for episode range(num_episodes):
state = env.reset()
done = False
while not done:
# Choose action based on epsilon-greedy
action = epsilon_greedy(q_table state, episode)
Take action and observe the next state and reward
next_state, reward, done = env.step(action)
# Update Q-table using the Q-learning update rule
q_value = q_table[state][action]
max_q_value = np.max(q_table[next_state])
q_table[state][action] += learning_rate * (reward + (discount_factor * max_q_value) - q_value)
state = next_state
return q_table
# Define epsilon-greedy policy
def epsilon_greedy(q_table, state, episode, epsilon=0.1):
if np.random.uniform() < epsilon * (1 / (episode + 1)):
# Explore by choosing a random action
action = np.random.choice(len(q_table[state]))
else:
# Exploit by choosing the action with maximum Q-value
action = np.argmax(q_table[state])
return action
# Define the environment
class Environment:
def __init__(self):
self.num_states = 6
self.num_actions = 2
self.transitions = {
0: {0: (2, -1), 1: (1, -1)},
1: {0: (3, -1), 1: (2, -1)},
2: {0: (4, -1), 1: (3, -1)},
3: {0: (5, -1), 1: (4, -1)},
4: {0: (5, 10), 1: (5, 0)},
5: {0: (5, 0), 1: (5, 0)}
}
def reset(self):
return 0
def step(self, action):
next_state, reward = self.transitions[state][action]
done = (next_state == self.num_states - 1)
return next_state, reward, done
# Run Q-learning
env = Environment()
q_table = q_learning(env, num_episodes=1000, learning_rate=0.1, discount_factor=0.9)
print("Q-table:")
print(q_table)
```
This code implements the Q-learning algorithm using an epsilon-greedy policy. The environment is a simple grid with 6 states and 2 actions. The Q-table is updated based on the observed rewards and transitions. After running the Q-learning algorithm, the final Q-table is printed. Feel free to modify the code to suit your specific problem.
阅读全文
相关推荐
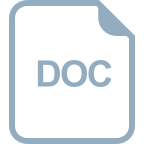
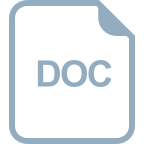
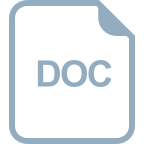

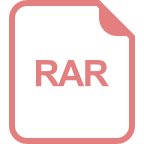
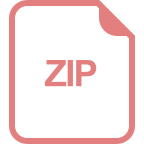
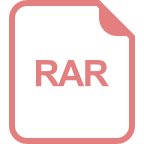
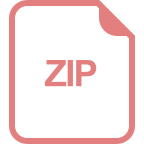
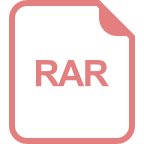







