python写入excel指定列
时间: 2023-09-16 19:14:06 浏览: 39
可以使用 Python 的 `openpyxl` 库来操作 Excel 文件,具体实现如下:
```python
import openpyxl
# 打开 Excel 文件
workbook = openpyxl.load_workbook('example.xlsx')
# 选择要操作的工作表
worksheet = workbook.active
# 写入数据到指定列
for i in range(1, 11):
worksheet.cell(row=i, column=2, value='Data {}'.format(i))
# 保存 Excel 文件
workbook.save('example.xlsx')
```
这里演示了如何将数据写入第 2 列(B 列)的前 10 行中。可以根据实际需求修改代码。
相关问题
python 写入excel指定列导入 df2.to_excel
你可以使用 pandas 库来实现将 DataFrame 写入到指定列的 Excel 文件中。具体的代码如下:
```python
import pandas as pd
# 读取 Excel 文件
df1 = pd.read_excel('file1.xlsx')
df2 = pd.read_excel('file2.xlsx')
# 将 df2 写入到 df1 的第2列中
with pd.ExcelWriter('file1.xlsx', mode='a') as writer:
df2.to_excel(writer, sheet_name='Sheet1', startcol=1, index=False, header=False)
```
其中,`startcol` 参数指定了写入的起始列,这里设为1表示从第2列开始写入(因为 Excel 的列是从1开始计数的)。`index=False` 和 `header=False` 参数表示不写入索引和列名。
python pandas写入excel指定列
要在Python Pandas中写入Excel指定列,您可以使用以下步骤:
1. 使用`pd.read_excel`函数读取Excel文件。
2. 准备好要写入的数据,将其插入到指定列中,可以使用`data.insert`方法将新列插入到特定位置。
3. 使用`DataFrame(data).to_excel`函数将修改后的数据写入Excel文件。
下面是一个示例代码,演示如何使用Python Pandas将数据写入Excel指定列:
```python
import pandas as pd
# 读取Excel文件
data = pd.read_excel('your_file.xlsx')
# 准备要写入的数据
infections = [1, 2, 3]
conts = [4, 5, 6]
clears = [7, 8, 9]
# 插入新列到指定位置
data.insert(2, 'infection', infections)
data.insert(3, 'cont', conts)
data.insert(4, 'clear', clears)
# 将修改后的数据写入Excel文件
data.to_excel('your_file.xlsx', sheet_name='Sheet1', index=False, header=True)
```
这样,您就可以将指定列的数据写入Excel文件了。
阅读全文
相关推荐







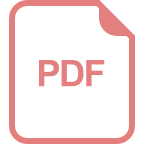
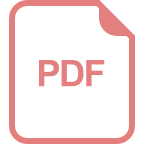