uni做小程序登录开发流程代码
时间: 2023-11-25 16:51:21 浏览: 75
Uni-app是一个跨平台的开发框架,可以快速构建小程序、APP、H5等多端应用。Uni-app小程序登录开发的代码流程如下:
1. 在登录页面的vue文件中,添加手机号/账号和密码输入框,以及登录按钮等组件:
```html
<template>
<view class="form">
<input type="text" placeholder="请输入手机号/账号" v-model="account" />
<input type="password" placeholder="请输入密码" v-model="password" />
<button type="primary" @click="login">登录</button>
</view>
</template>
```
2. 在登录页面的js文件中,编写登录事件的处理函数,用于向后台服务器发送登录请求:
```javascript
export default {
data() {
return {
account: '',
password: ''
};
},
methods: {
login() {
uni.request({
url: 'https://example.com/login',
method: 'POST',
data: {
account: this.account,
password: this.password
},
success: (res) => {
if (res.statusCode === 200) {
uni.setStorageSync('token', res.data.token);
uni.navigateTo({
url: '../index/index'
});
} else {
uni.showToast({
title: '登录失败',
icon: 'none'
});
}
},
fail: () => {
uni.showToast({
title: '登录失败',
icon: 'none'
});
}
});
}
}
};
```
3. 在后台服务器中,编写登录接口的代码,用于验证用户输入的手机号/账号和密码是否正确,并生成token返回给小程序:
```python
@app.route('/login', methods=['POST'])
def login():
account = request.json.get('account')
password = request.json.get('password')
user = User.query.filter_by(account=account).first()
if user is not None and user.verify_password(password):
token = user.generate_auth_token()
return jsonify({'token': token.decode('ascii')})
return jsonify({'error': 'invalid account or password'}), 401
```
4. 在Uni-app的main.js文件中,编写全局请求拦截器,用于在发送请求前添加token:
```javascript
uni.addInterceptor('request', {
success: (res) => {
if (res.statusCode === 401) {
uni.showToast({
title: '请先登录',
icon: 'none'
});
uni.navigateTo({
url: '../login/login'
});
}
}
});
uni.addInterceptor('request', {
success: (res) => {
var token = uni.getStorageSync('token');
if (token) {
res.header.Authorization = 'Bearer ' + token;
}
}
});
```
这样,当用户输入手机号/账号和密码后,Uni-app会向后台服务器发送登录请求,在服务器端验证通过后,生成一个token并返回给小程序。小程序将token保存在本地缓存中,在后续请求时添加到请求头中进行验证,以保证用户的登录状态。
阅读全文
相关推荐
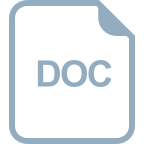
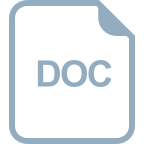
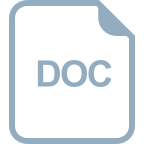

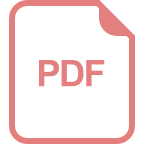
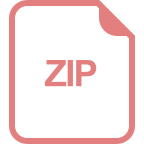
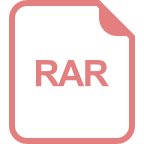
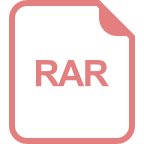
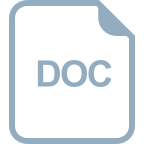
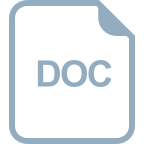
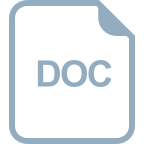
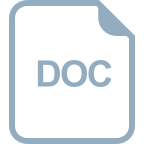






