如何使用Confluence API获取网页表格里内容,请写出Python代码
时间: 2023-08-31 12:36:14 浏览: 338

Python库 | foliantcontrib.confluence-0.6.8.tar.gz
### 回答1:
答:可以使用Python库confluence-python来获取Confluence API中表格的内容,例如:from confluence import Confluenceconf = Confluence()table_content = conf.get_table_content(page_id, table_id)print(table_content)
### 回答2:
要使用Confluence API获取网页表格里的内容,可以使用Python编写代码来实现。首先,需要安装 `requests` 模块来发送HTTP请求,并安装 `json` 模块来解析返回的JSON数据。
以下是一个使用Confluence API获取网页表格内容的Python代码示例:
```python
import requests
import json
# 设置Confluence的URL和页面ID
confluence_url = "https://<your-confluence-url>/wiki/rest/api/content/<your-page-id>"
# 发送HTTP GET请求获取页面内容
response = requests.get(confluence_url)
# 检查响应状态码
if response.status_code == 200:
# 解析JSON数据
data = json.loads(response.text)
# 获取表格内容
table_content = ""
for table_row in data["body"]["storage"]["value"]:
# 检查是否包含表格
if table_row.get("table"):
for row in table_row["table"]["rows"]:
for cell in row["cells"]:
table_content += cell["value"] + "\t" # 将单元格内容以制表符分隔
print(table_content)
else:
print("Failed to retrieve page content.")
```
在上述代码中,需要将 `<your-confluence-url>` 替换为你的Confluence URL,`<your-page-id>` 替换为你要获取表格内容的页面的ID。运行代码后,它将输出表格内容,并将每个单元格的内容以制表符分隔。
请注意,你还需要进行身份验证和授权才能访问Confluence API。具体的身份验证和授权过程将因你的环境和配置而异。你可以参考Confluence API文档以了解如何进行身份验证和授权,并在代码中添加相应的步骤。
### 回答3:
要使用Confluence API获取网页表格里的内容,可以使用Python编程语言,并使用requests库来发送HTTP请求。
首先,需要安装requests库,使用以下命令进行安装:
```
pip install requests
```
接下来,可以使用以下代码来获取Confluence页面的内容,包括网页表格的内容:
```python
import requests
# 定义Confluence页面的URL
url = 'https://your-confluence-url/rest/api/content/PAGE_ID?expand=body.view'
# 定义用于身份验证的用户名和密码
username = 'your-username'
password = 'your-password'
# 发送GET请求获取Confluence页面的内容
response = requests.get(url, auth=(username, password))
# 检查请求是否成功
if response.status_code == 200:
data = response.json()
# 获取页面正文内容
body = data['body']['view']['value']
# 在正文内容中查找表格内容
start_index = body.find('<table>')
end_index = body.find('</table>')
# 提取表格内容
table_content = body[start_index:end_index + len('</table>')]
# 打印表格内容
print(table_content)
else:
print('Failed to retrieve Confluence page. Status code:', response.status_code)
```
请注意替换以下变量的值:
- 'https://your-confluence-url':替换为您的Confluence实例的URL。
- 'PAGE_ID':替换为您要获取内容的页面的ID。
- 'your-username':替换为您的Confluence用户名。
- 'your-password':替换为您的Confluence密码。
这段代码将发送一个GET请求,获取Confluence页面的内容,并从页面正文中提取表格内容。
阅读全文
相关推荐
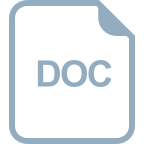
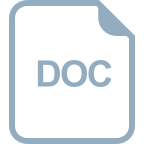

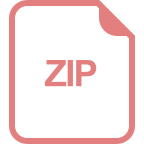













