编写一个有关生产者和消费者的程序:每个生产者每次生产一个产品存入仓库,每个消费者 每次从仓库中取出一个产品进行消费,仓库大小有限,每次只能有一个生产者或消费者访问 仓库。要求:采用信号量机制。 C语言
时间: 2024-09-12 09:02:31 浏览: 55
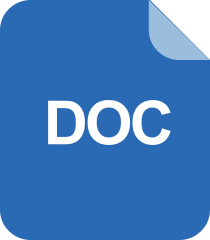
实现生产者消费者问题

在C语言中,我们可以使用互斥锁(mutex)和条件变量(condition variable)结合信号量(semaphore)来实现生产者-消费者模型。下面是一个简单的示例:
```c
#include <stdio.h>
#include <pthread.h>
#define MAX_CAPACITY 5
#define SEMAPHORE_PRODUCER 1
#define SEMAPHORE_CONSUMER 2
typedef struct {
int capacity;
int items;
int semaphore_prod;
int semaphore_cons;
int buffer[MAX_CAPACITY];
} ProdCons;
void* producer_func(void* arg) {
ProdCons* pc = (ProdCons*)arg;
int item = 0;
while (1) {
// 生产者获取生产权限
pthread_mutex_lock(&pc->semaphore_prod);
if (pc->items < pc->capacity) { // 如果仓库未满
pc->items++;
printf("Producer produced item %d, total: %d\n", item++, pc->items);
pthread_cond_signal(&pc->semaphore_cons); // 醒通知消费者
} else {
pthread_cond_wait(&pc->semaphore_prod, &pc->semaphore_cons); // 仓库满了,等待消费者处理
}
pthread_mutex_unlock(&pc->semaphore_prod);
}
}
void* consumer_func(void* arg) {
ProdCons* pc = (ProdCons*)arg;
while (1) {
// 消费者获取消费权限
pthread_mutex_lock(&pc->semaphore_cons);
if (pc->items > 0) { // 如果仓库中有商品
pc->items--;
printf("Consumer consumed item %d, total: %d\n", pc->buffer[pc->items], pc->items);
} else {
pthread_cond_wait(&pc->semaphore_cons, &pc->semaphore_prod); // 仓库空了,等待生产者填充
}
pthread_mutex_unlock(&pc->semaphore_cons);
}
}
int main() {
ProdCons prodCons = {
.capacity = MAX_CAPACITY,
.items = 0,
.semaphore_prod = PTHREAD_MUTEX_INITIALIZER,
.semaphore_cons = PTHREAD_MUTEX_INITIALIZER,
};
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer_func, &prodCons);
pthread_create(&consumer_thread, NULL, consumer_func, &prodCons);
// 等待线程结束
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
```
在这个例子中,我们创建了一个`ProdCons`结构来保存生产者-消费者共享的数据,并初始化了两个互斥锁(semaphore_prod 和 semaphore_cons)。生产者负责增加库存并唤醒消费者,而消费者则负责消耗库存并在必要时等待。
阅读全文
相关推荐
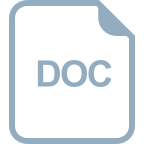
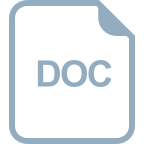
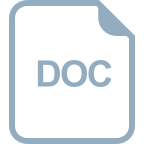
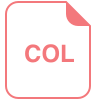
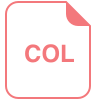
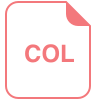
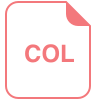
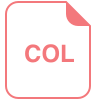
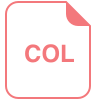
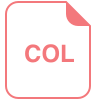
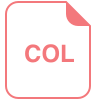
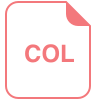
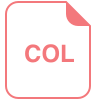
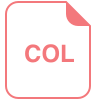
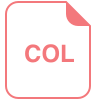
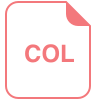
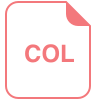
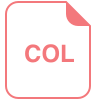