代码实现java多线程压缩文件
时间: 2023-10-30 18:08:05 浏览: 64
可以使用Java的多线程和压缩文件相关的类来实现多线程压缩文件。以下是一个简单的实现方法:
1. 首先,创建一个实现Runnable接口的类,用于执行压缩文件的任务。在这个类中,我们可以使用Java的ZipOutputStream类来压缩文件。
2. 在主程序中,创建一个线程池,用于执行多个任务。然后,将每个任务添加到线程池中。
3. 在添加任务之前,我们需要先将要压缩的文件列表传递给每个任务。这可以通过构造函数或setter方法来完成。
4. 在每个任务中,我们可以使用Java的CountDownLatch类来等待所有线程完成任务。这可以确保所有文件都已经被压缩。
5. 最后,我们可以将所有压缩后的文件合并成一个文件。这可以使用Java的ZipFileUtils类来完成。
下面是一个简单的示例代码:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipTask implements Runnable {
private List<File> fileList;
private String outputZipFile;
private CountDownLatch latch;
public ZipTask(List<File> fileList, String outputZipFile, CountDownLatch latch) {
this.fileList = fileList;
this.outputZipFile = outputZipFile;
this.latch = latch;
}
@Override
public void run() {
try {
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(outputZipFile));
for (File file : fileList) {
addFileToZip(file, zos);
}
zos.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
latch.countDown();
}
}
private void addFileToZip(File file, ZipOutputStream zos) throws IOException {
FileInputStream fis = new FileInputStream(file);
ZipEntry zipEntry = new ZipEntry(file.getName());
zos.putNextEntry(zipEntry);
byte[] bytes = new byte[1024];
int length;
while ((length = fis.read(bytes)) >= 0) {
zos.write(bytes, 0, length);
}
zos.closeEntry(); fis.close();
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
List<File> fileList = ...; // 获取要压缩的文件列表
int numThreads = ...; // 指定线程数
String outputZipFile = ...; // 指定输出文件名
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
CountDownLatch latch = new CountDownLatch(numThreads);
int numFilesPerThread = fileList.size() / numThreads;
int startIndex = 0;
int endIndex = numFilesPerThread;
for (int i = 0; i < numThreads; i++) {
if (i == numThreads - 1) {
endIndex = fileList.size();
}
List<File> subList = fileList.subList(startIndex, endIndex);
executor.execute(new ZipTask(subList, "output" + i + ".zip", latch));
startIndex = endIndex;
endIndex += numFilesPerThread;
}
latch.await();
// 合并所有压缩文件
ZipFileUtils.mergeZipFiles(outputZipFile, "output*.zip");
executor.shutdown();
}
}
```
相关推荐
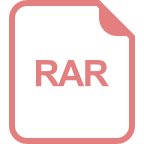
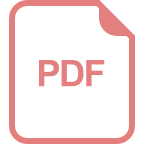














