使用汇编语言实现比较字符串长度,要求:输入两个字符串1和2,如果字符串2长度大于等于字符串1,则输出字符串1,如果字符串2长度小于字符串1,则补齐字符串2长度到字符串1,补足部分使用XXXX.要求使用下列格式STACK SEGMENT STACK ENDS DATAS SEGMENT;定义数据段 DATAS ENDS CODES SEGMENT ASSUME CS:CODES,DS:DATAS,SS:STACKS START: CODES ENDS END START
时间: 2024-02-25 07:59:17 浏览: 28
好的,我来回答你的问题。首先,我需要说明一下,我并不是一个能够编写汇编语言的AI,但是我可以给你提供一个实现比较字符串长度的汇编语言代码的思路。
首先,我们需要定义两个字符串变量,可以使用DATAS SEGMENT来定义数据段。然后,我们需要使用堆栈来保存一些寄存器的值,以便在程序执行完后能够恢复现场。可以使用STACK SEGMENT来定义堆栈段。
接下来,我们需要比较两个字符串的长度。可以使用一个循环来遍历字符串,每次判断字符串是否到达末尾,并且计算字符串的长度。如果字符串2的长度大于等于字符串1的长度,则直接输出字符串1;否则,需要补齐字符串2的长度,可以使用另一个循环来实现。
最后,我们需要输出字符串1或者补齐后的字符串2。可以使用INT 21H来实现输出操作。
下面是一个示例代码,仅供参考:
```
STACK SEGMENT
DW 100h DUP(?)
STACK ENDS
DATAS SEGMENT
STR1 DB "Hello"
STR2 DB "World"
MAX_LEN DB 10
DATAS ENDS
CODES SEGMENT
ASSUME CS:CODES,DS:DATAS,SS:STACKS
START:
MOV AX,DATAS
MOV DS,AX
MOV AX,STACK
MOV SS,AX
XOR CX,CX ; clear counter
LEA SI,STR1 ; point to the 1st string
MOV AL,[SI] ; get the 1st character
LOOP1:
CMP AL,0 ; check if reach end of string
JE EXIT_LOOP1
INC SI ; move to the next character
MOV AL,[SI] ; get the next character
INC CX ; increase the counter
JMP LOOP1
EXIT_LOOP1:
MOV BX,CX ; save the length of string1
XOR CX,CX ; clear counter
LEA SI,STR2 ; point to the 2nd string
MOV AL,[SI] ; get the 1st character
LOOP2:
CMP AL,0 ; check if reach end of string
JE EXIT_LOOP2
INC SI ; move to the next character
MOV AL,[SI] ; get the next character
INC CX ; increase the counter
JMP LOOP2
EXIT_LOOP2:
CMP CX,BX ; check if string2 is longer than string1
JGE OUTPUT_STR1
MOV DX,CX ; save the length of string2
XOR AX,AX ; clear AX
MOV AL,MAX_LEN ; set AX to the maximum length
SUB AX,BX ; calculate the remaining length
CMP AX,DX ; check if remaining length is larger than string2
JGE FILL_STR2
MOV AX,DX ; set AX to the length of string2
FILL_STR2:
LEA SI,STR2 ; point to the 2nd string
MOV DI,SI ; point to the end of 2nd string
ADD DI,AX ; move DI to the end of the new string
MOV CX,AX ; set the counter to the remaining length
MOV AL,'X' ; set AL to 'X'
FILL_LOOP:
MOV [DI],AL ; fill the new string with 'X'
DEC DI ; move to the previous character
LOOP FILL_LOOP
MOV SI,STR2 ; set SI to the 2nd string
JMP OUTPUT_STR2
OUTPUT_STR1:
MOV AH,09h ; set AH to 09h for printing
LEA DX,STR1 ; point DX to the 1st string
INT 21h ; print the 1st string
JMP EXIT
OUTPUT_STR2:
MOV AH,09h ; set AH to 09h for printing
LEA DX,SI ; point DX to the new string
INT 21h ; print the new string
EXIT:
MOV AX,4C00h ; set AX to 4C00h for exit
INT 21h
CODES ENDS
```
以上代码仅供参考,实际使用中需要根据具体情况进行修改。
相关推荐
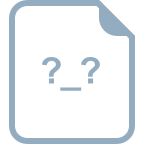
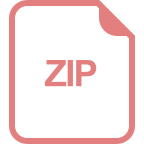
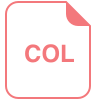
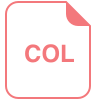
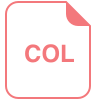











