STM32ADC串口输出
时间: 2024-01-01 18:04:35 浏览: 80
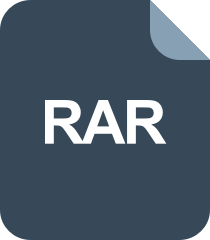
ADC01_uart.rar
要在STM32上使用ADC并将其值通过串口输出,可以按照以下步骤进行:
1. 配置ADC通道和采样时间
2. 配置USART串口
3. 在ADC转换完成后,将其值转换为字符串并通过USART发送
下面是一个简单的示例代码:
```
#include "stm32f4xx.h"
#include <stdio.h>
void ADC_Config(void);
void USART_Config(void);
void USART_SendString(char* s);
int main(void)
{
ADC_Config();
USART_Config();
while (1)
{
// 开始ADC转换
ADC_SoftwareStartConv(ADC1);
// 等待转换完成
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
// 将ADC值转换为字符串
uint16_t adc_value = ADC_GetConversionValue(ADC1);
char buffer[10];
sprintf(buffer, "%d\r\n", adc_value);
// 发送字符串到USART
USART_SendString(buffer);
// 延时一段时间
for (int i = 0; i < 1000000; i++);
}
}
void ADC_Config(void)
{
ADC_InitTypeDef ADC_InitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
// 使能GPIOA和ADC1时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
// 配置PA0为模拟输入
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0; GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// 配置ADC1
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = DISABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
// 配置ADC通道0和采样时间
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_84Cycles);
// 使能ADC1
ADC_Cmd(ADC1, ENABLE);
}
void USART_Config(void)
{
USART_InitTypeDef USART_InitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
// 使能GPIOA和USART2时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
// 配置PA2为USART2的TX引脚
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// 将PA2映射到USART2的TX引脚
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
// 配置USART2
USART_InitStruct.USART_BaudRate = 115200;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx;
USART_Init(USART2, &USART_InitStruct);
// 使能USART2
USART_Cmd(USART2, ENABLE);
}
void USART_SendString(char* s)
{
while (*s)
{
while (USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
USART_SendData(USART2, *s++);
}
}
相关问题:
阅读全文
相关推荐
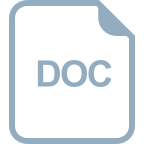
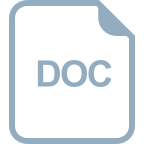
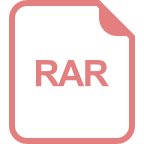
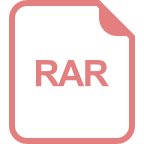
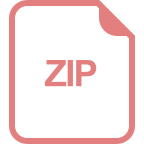
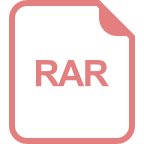
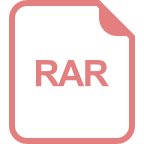
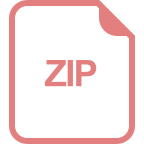
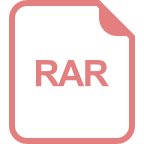
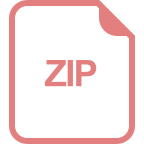
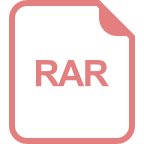
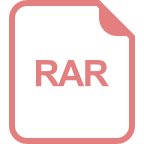
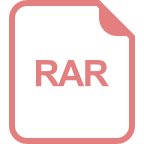
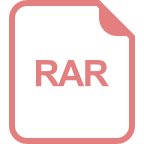
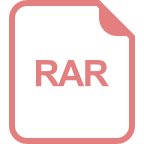
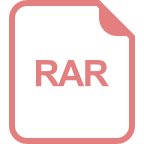