帮我写一个完整的这个图片查看器的程序
时间: 2024-09-22 08:07:15 浏览: 29
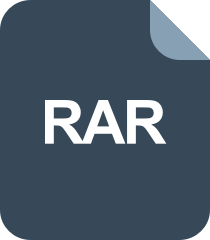
Win10图片查看器bat、win7图片查看器
由于编写一个完整的Java Swing应用程序涉及到很多代码,这里我将给出一个简化版的基本框架,你可以基于此构建一个基本的图片查看器。首先确保已导入必要的库:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
public class ImageViewer extends JFrame implements ActionListener {
private JButton openButton, prevButton, nextButton;
private JLabel imageView;
private ArrayList<String> imagesList;
private int currentIndex = 0;
public ImageViewer() {
// 设置窗口属性
setTitle("图片查看器");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
// 初始化控件和成员变量
initComponents();
// 显示第一张图片
displayCurrentImage(imagesList.get(currentIndex));
}
private void initComponents() {
JPanel panel = new JPanel(new BorderLayout());
imagesList = new ArrayList<>();
openButton = new JButton("打开");
openButton.addActionListener(this);
prevButton = new JButton("上一张");
prevButton.addActionListener(this);
nextButton = new JButton("下一张");
nextButton.addActionListener(this);
imageView = new JLabel();
openButton.setBounds(10, 10, 100, 30);
prevButton.setBounds(120, 10, 70, 30);
nextButton.setBounds(210, 10, 70, 30);
imageView.setBounds(30, 40, 640, 480);
panel.add(openButton, BorderLayout.WEST);
panel.add(prevButton, BorderLayout.NORTH);
panel.add(nextButton, BorderLayout.EAST);
panel.add(imageView, BorderLayout.CENTER);
setContentPane(panel);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == openButton) {
showFileChooser();
} else if (e.getSource() == prevButton) {
changeImage(-1);
} else if (e.getSource() == nextButton) {
changeImage(1);
}
}
private void showFileChooser() {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File selectedFile = fileChooser.getSelectedFile();
try {
String filePath = selectedFile.getAbsolutePath();
if (!imagesList.contains(filePath)) {
imagesList.add(filePath);
Collections.sort(imagesList); // 如果有需求,可以排序
}
updateImageList();
displayCurrentImage(filePath);
} catch (IOException ioe) {
JOptionPane.showMessageDialog(this, "文件打开失败:" + ioe.getMessage(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
private void updateImageList() {
// 更新显示图片的数量等信息
}
private void displayCurrentImage(String imagePath) {
try {
ImageIcon imgIcon = new ImageIcon(imagePath);
imageView.setIcon(imgIcon);
if (currentIndex == 0) {
JOptionPane.showMessageDialog(this, "这是第一张图片");
} else if (currentIndex == imagesList.size() - 1) {
JOptionPane.showMessageDialog(this, "这是最后一张图片");
}
} catch (IOException ioe) {
System.out.println("加载图片失败:" + ioe.getMessage());
}
}
private void changeImage(int delta) {
currentIndex += delta;
if (currentIndex < 0) {
currentIndex = 0;
} else if (currentIndex >= imagesList.size()) {
currentIndex = imagesList.size() - 1;
}
displayCurrentImage(imagesList.get(currentIndex));
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
ImageViewer viewer = new ImageViewer();
viewer.setVisible(true);
});
}
}
```
这个代码示例包含了一个简单的图片查看器,用户可以选择图片,然后浏览上一张、下一张,并看到当前图片是否是第一张或最后一张。注意,为了保持简洁,这里没有处理文件打开失败的情况以及显示图片数量的信息。在实际应用中,你可能还需要完善错误处理和用户体验。
阅读全文
相关推荐
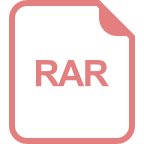
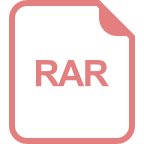
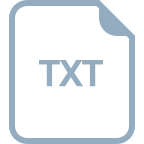
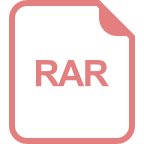
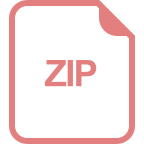
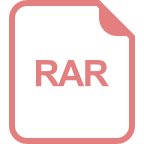
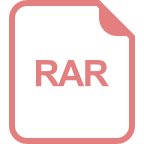
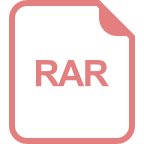
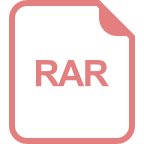
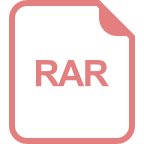
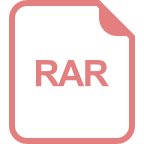
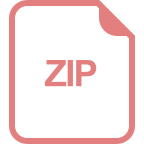
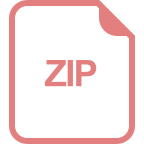
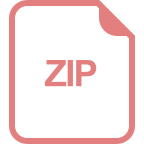
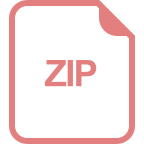
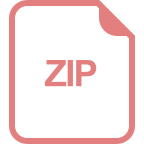