用java写一个完整的一定能够跑通的MapReduce根据统计文档中buy最多的前10个商品的id(item_id)和前10个fav类型的商品id(item_id)
时间: 2024-09-23 13:14:34 浏览: 43
要使用Java编写一个可以运行的Hadoop MapReduce程序来处理如`cookie.txt`这样的日志文件,并找出其中`buy`操作次数最多和`fav`操作次数最多的前十件商品ID (`item_id`),我们需要定义Mapper类、Reducer类以及驱动主函数。下面是一个基本实现示例:
### Mapper类
此Mapper将从输入键值对中提取出商品ID (`item_id`) 和行为类型 (如`buy`, `fav`) 并为每种行为输出相应的键值对。
```java
import java.io.IOException;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class ItemBehaviorMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] parts = value.toString().split(",");
if (parts.length >= 3 && ("buy".equals(parts[2]) || "fav".equals(parts[2]))) {
word.set(parts[1]);
context.write(word, one);
}
}
}
```
### Reducer类
这个Reducer负责汇总每个商品的行为计数,并分别保存`buy`与`fav`的结果。
```java
import java.io.IOException;
import java.util.TreeMap;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class TopItemsReducer extends Reducer<Text,IntWritable,Text,IntWritable> {
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
// 这里简化了逻辑,实际应用中可能需要更复杂的处理以获取Top N结果
context.write(key, new IntWritable(sum));
}
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
super.cleanup(context);
// 实现收集并排序top 10的功能
}
}
```
### 主程序
主程序设置作业参数,并启动任务。
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class TopItemStats {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "top item stats");
job.setJarByClass(TopItemStats.class);
job.setMapperClass(ItemBehaviorMapper.class);
job.setReducerClass(TopItemsReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
请注意,以上代码片段仅作为基础框架提供,并未完全实现top 10排名功能;具体实现时还需要考虑如何在Reducer端或后续阶段进行数据的排序及筛选。此外,在真实环境中部署此类应用程序之前,还需配置好Hadoop环境,并确保所有依赖正确无误。
阅读全文
相关推荐
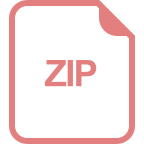
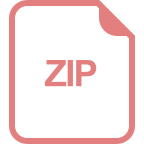
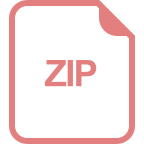

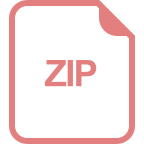
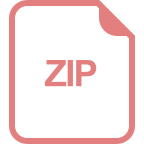
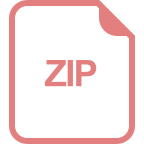
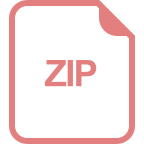
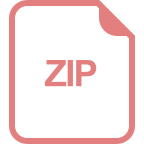
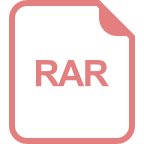
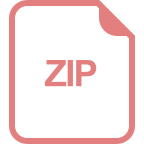
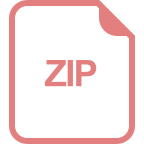
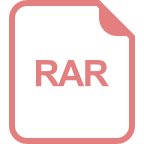
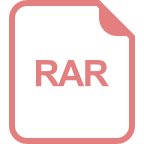
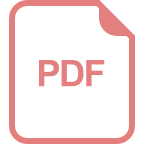
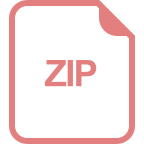
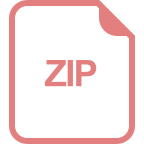
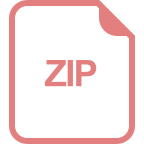
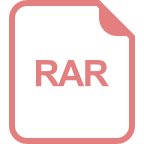
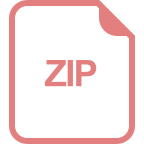