Qt中如何将按钮设置为可移动的
时间: 2024-04-10 14:33:05 浏览: 138
在Qt中,可以通过设置按钮的`setMovable()`函数来将按钮设置为可移动的。以下是一个示例代码:
```cpp
#include <QtWidgets>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget window;
QVBoxLayout layout;
QPushButton button("可移动按钮");
// 设置按钮为可移动
button.setMovable(true);
layout.addWidget(&button);
window.setLayout(&layout);
window.show();
return app.exec();
}
```
在上述示例中,我们创建了一个可移动的按钮,通过`setMovable(true)`将按钮设置为可移动。然后将按钮添加到布局中,并将布局设置为窗口的布局。最后显示窗口并启动应用程序事件循环。
当你运行该代码时,你将看到一个可移动的按钮。你可以点击并拖动按钮来移动它在窗口中的位置。
相关问题
qt使用QGridLayout将按钮设置为可移动
您可以使用QGridLayout和QPushButton来实现将按钮设置为可移动。首先,创建一个QGridLayout布局,并将其设置为窗口的主布局。
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QGridLayout, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建一个窗口部件并设置布局
central_widget = QWidget(self)
self.setCentralWidget(central_widget)
grid_layout = QGridLayout(central_widget)
central_widget.setLayout(grid_layout)
# 创建可移动的按钮
button1 = QPushButton("Button 1", self)
button2 = QPushButton("Button 2", self)
# 将按钮添加到布局中
grid_layout.addWidget(button1, 0, 0)
grid_layout.addWidget(button2, 0, 1)
# 设置按钮为可移动
button1.setCheckable(True)
button2.setCheckable(True)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上述示例中,我们创建了一个主窗口,并将一个QGridLayout作为主布局。然后,我们创建了两个QPushButton按钮,并将它们添加到布局中的第一行。通过将按钮的`setCheckable(True)`方法调用,我们将按钮设置为可移动的。当按钮被按下时,它将保持按下状态,当再次按下时,它将恢复正常状态。
这样,您就可以使用QGridLayout将按钮设置为可移动。您可以根据需要添加更多的按钮,并在布局中进行自定义。
QT 中如何设置一个网格布局,布局里有 2*4 8个图标按钮,并让每个按钮边缘为弧形,按钮上显示一张图片边缘也为弧形。每个按钮下面都有一个文字框。同时实现可单独拖拽和移动每个按钮
在Qt中,你可以使用`QGridLayout`来实现一个网格布局,并将图标按钮和文字框放置在其中。为了让按钮和按钮上的图片边缘为弧形,并实现可拖拽和移动的功能,你可以使用`QGraphicsEffect`和事件过滤器。
下面是一个示例代码,演示了如何实现这个布局:
```cpp
#include <QApplication>
#include <QWidget>
#include <QGridLayout>
#include <QPushButton>
#include <QLabel>
#include <QGraphicsDropShadowEffect>
#include <QGraphicsPixmapItem>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QMouseEvent>
class DraggableButton : public QPushButton
{
public:
DraggableButton(QWidget *parent = nullptr) : QPushButton(parent)
{
setAcceptDrops(true);
setStyleSheet("QPushButton {border-radius: 10px;}");
QGraphicsDropShadowEffect *effect = new QGraphicsDropShadowEffect;
effect->setBlurRadius(10);
effect->setOffset(0);
effect->setColor(Qt::gray);
setGraphicsEffect(effect);
QLabel *label = new QLabel(this);
label->setAlignment(Qt::AlignCenter);
label->setText("Text");
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(label);
setLayout(layout);
}
protected:
void mousePressEvent(QMouseEvent *event) override
{
if (event->button() == Qt::LeftButton) {
startPos = event->pos();
}
QPushButton::mousePressEvent(event);
}
void mouseMoveEvent(QMouseEvent *event) override
{
if (event->buttons() & Qt::LeftButton) {
int distance = (event->pos() - startPos).manhattanLength();
if (distance >= QApplication::startDragDistance()) {
QDrag *drag = new QDrag(this);
QMimeData *mimeData = new QMimeData;
drag->setMimeData(mimeData);
drag->exec();
}
}
QPushButton::mouseMoveEvent(event);
}
private:
QPoint startPos;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget widget;
QGridLayout *gridLayout = new QGridLayout(&widget);
QGraphicsScene scene;
QGraphicsView view(&scene);
view.setRenderHint(QPainter::Antialiasing);
for (int row = 0; row < 2; ++row) {
for (int col = 0; col < 4; ++col) {
DraggableButton *button = new DraggableButton(&widget);
QPixmap pixmap(":/path/to/image");
QGraphicsPixmapItem *pixmapItem = scene.addPixmap(pixmap);
pixmapItem->setShapeMode(QGraphicsPixmapItem::BoundingRectShape);
pixmapItem->setPixmap(pixmap.scaled(80, 80, Qt::KeepAspectRatio, Qt::SmoothTransformation));
QGraphicsDropShadowEffect *effect = new QGraphicsDropShadowEffect;
effect->setBlurRadius(10);
effect->setOffset(0);
effect->setColor(Qt::gray);
pixmapItem->setGraphicsEffect(effect);
gridLayout->addWidget(button, row, col);
}
}
widget.setLayout(gridLayout);
widget.show();
return app.exec();
}
```
在这个示例中,我们创建了一个名为 "DraggableButton" 的自定义QPushButton类,用于实现可拖拽和移动的按钮。我们重写了鼠标事件函数`mousePressEvent`和`mouseMoveEvent`,以实现按钮的拖拽功能。我们还使用了`QGraphicsDropShadowEffect`来设置按钮和按钮上的图片边缘为弧形。
在主函数中,我们创建了一个QWidget作为窗口,并使用QGridLayout来实现网格布局。然后,我们使用QGraphicsScene和QGraphicsView来显示每个按钮上的图片,并设置图片边缘为弧形。
最后,我们将自定义的DraggableButton添加到网格布局中,并将QWidget显示出来。
请注意,示例中的图片路径需要替换为你自己的图片路径。
希望这个示例能对你有所帮助!如果有任何问题,请随时提问。
阅读全文
相关推荐
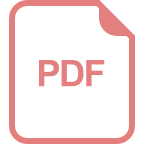
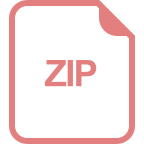
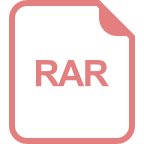
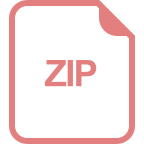
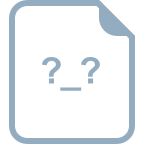
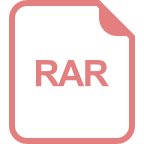
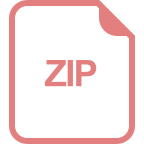
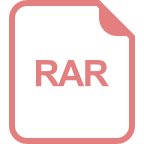
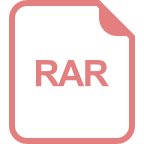





