python爬虫酷狗会员音乐
时间: 2024-08-12 22:01:41 浏览: 51
Python爬虫用于抓取网站数据,包括酷狗音乐等在线平台的信息。针对酷狗会员音乐这类需要登录权限的内容,你需要使用一些技术来处理:
1. **模拟登录**:首先,通过requests库发送GET请求获取登录页面的cookie或session信息,然后构造POST请求携带这些信息尝试登录。
2. **使用Selenium**:如果网站有复杂的JavaScript交互,可以考虑使用浏览器自动化工具Selenium配合WebDriver,它能模拟真实用户行为。
3. **处理反爬策略**:酷狗可能会有IP限制、验证码识别、动态加载内容等反爬机制,需要适当设置延时、代理IP、OCR识别等技巧来绕过。
4. **分析网页结构**:使用BeautifulSoup、PyQuery等库解析HTML文档,提取所需音乐信息,如歌曲名、歌手、链接等。
5. **保存数据**:将抓取的数据存储到本地文件、数据库或是云服务,以便后续分析或分享。
然而,需要注意的是,在实际操作中,遵守网站的Robots协议,并尊重版权法律是非常重要的。如果你打算进行大规模的爬虫项目,最好先了解并申请相应的API授权,而不是直接爬取网站内容。
相关问题
python爬虫酷狗音乐
你可以使用Python来编写爬虫程序来获取酷狗音乐的数据。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头信息,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
# 发送GET请求获取网页内容
url = 'http://www.kugou.com/yy/rank/home/1-8888.html?from=homepage'
response = requests.get(url, headers=headers)
html = response.text
# 使用BeautifulSoup解析html
soup = BeautifulSoup(html, 'html.parser')
# 找到包含歌曲信息的元素
songs = soup.find_all('li', class_='pc_temp_songlist')
# 遍历歌曲信息并获取相关数据
for song in songs:
# 获取歌曲名称
name = song.find('a', class_='pc_temp_songname').text.strip()
# 获取歌手名称
singer = song.find('a', class_='pc_temp_singername').text.strip()
# 打印歌曲信息
print(f"歌曲:{name},歌手:{singer}")
```
这段代码使用了requests库发送GET请求,并使用BeautifulSoup库解析HTML页面。你可以根据自己的需求进一步处理获取到的数据。请注意,爬取网站的数据需要遵守相关的法律法规,确保你的行为合法合规。
python爬虫酷狗音乐top20
以下是爬取酷狗音乐TOP20的Python代码:
```python
import requests
from bs4 import BeautifulSoup
import csv
# 请求头信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 获取HTML页面
def get_html(url):
try:
r = requests.get(url, headers=headers)
r.raise_for_status()
r.encoding = r.apparent_encoding
return r.text
except:
return ""
# 解析HTML页面
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
ranks = soup.find_all('span', class_='pc_temp_num')
titles = soup.find_all('a', class_='pc_temp_songname')
times = soup.find_all('span', class_='pc_temp_time')
for rank, title, time in zip(ranks, titles, times):
rank = rank.get_text().strip()
title = title.get_text().split('-')[1].strip()
author = title.get_text().split('-')[0].strip()
time = time.get_text().strip()
yield {'rank': rank, 'title': title, 'author': author, 'time': time}
# 保存数据到CSV文件
def save_to_csv(data):
with open('music.csv', 'w', newline='', encoding='utf-8-sig') as f:
writer = csv.writer(f)
writer.writerow(['排名', '歌曲名称', '歌手', '时长'])
for item in data:
writer.writerow([item['rank'], item['title'], item['author'], item['time']])
# 主函数
def main():
url = 'https://www.kugou.com/yy/rank/home/1-8888.html?from=rank'
html = get_html(url)
data = parse_html(html)
save_to_csv(data)
if __name__ == '__main__':
main()
```
相关推荐
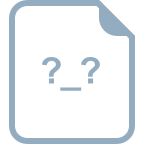
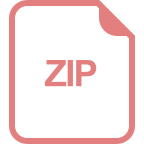












