3、 编写一段程序,使用系统调用fork()来创建一个子进程。子进程通过系统调用exec()更换自己的执行代码,新的代码显示“new program.”后,调用exit()结束。父进程则调用waitpid()等待子进程结束,并在子进程结束后,显示子进程的标识符然后正常结束。
时间: 2024-09-27 20:18:13 浏览: 73
在Unix/Linux环境中,你可以使用以下C语言代码来完成这个任务:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main(int argc, char *argv[]) {
// Fork a child process
pid_t pid = fork();
if (pid == 0) { // Child process
printf("Child process created with PID %d\n", getpid());
// Execute a new program (e.g., an echo command)
execvp("/bin/sh", "-c", "echo 'new program.'");
// If execvp fails, we reach here; we should never get here though
perror("Error executing the program");
exit(1);
} else if (pid > 0) { // Parent process
printf("Parent process started with PID %d\n", getpid());
// Wait for the child to finish
int status;
waitpid(pid, &status, 0);
printf("Child process %d terminated with status %d\n", pid, WEXITSTATUS(status));
} else { // Error in fork()
perror("Fork failed");
exit(1);
}
return 0;
}
```
在这个程序中,`fork()`函数用于创建子进程,如果返回值为0,则表示当前进程是新创建的子进程;非零值表示原进程。子进程中,我们调用`execvp()`替换自身为一个新的命令行解释器,执行指定的`echo`命令,显示"new program."。`waitpid()`函数在父进程部分用来等待子进程结束,获取其退出状态。
运行这个程序,你会看到父进程先输出,然后是子进程的输出,以及子进程结束后的信息。请注意,实际执行`/bin/sh -c "echo 'new program.'"`时,你需要有相应的权限,并确保`echo`命令在目标系统上可用。
阅读全文
相关推荐







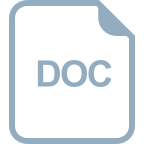


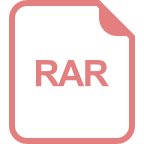







