c语言打印ascll码
时间: 2024-10-04 11:03:59 浏览: 31
在C语言中,你可以通过特定的方式打印ASCII码。ASCII(American Standard Code for Information Interchange,美国信息交换标准代码)是一种字符编码系统,将每个字符映射到一个唯一的整数值。以下是两个常见的方法:
1. 使用printf函数:
```c
#include <stdio.h>
int main() {
char ch = 'A'; // 或者任何其他字符
printf("ASCII code of character '%c' is %d\n", ch, ch);
return 0;
}
```
这里的`%d`会将字符的ASCII值作为十进制数输出。
2. 使用 putchar 函数:
```c
#include <stdio.h>
int main() {
char ch = 'A';
int ascii_value = (int)ch; // 将字符转换为整型
printf("ASCII value of character: %i\n", ascii_value);
putchar(ch); // 输出字符
return 0;
}
```
这两个例子都会打印出给定字符的ASCII码及其对应的字符。
相关问题
C语言用ascll码打印字符
C语言中,ASCII码(American Standard Code for Information Interchange,美国信息交换标准代码)是用来表示字符的标准编码。如果你想在控制台上打印字符,你可以通过将字符对应的ASCII值转换为其二进制形式,然后用printf函数输出。
例如,如果你想打印字符 'A',其ASCII值是65,你可以这样做:
```c
#include <stdio.h>
int main() {
char character = 'A';
int ascii_value = (int)character; // 将字符转换为整数
printf("The ASCII value of '%c' is %d.\n", character, ascii_value);
// 如果需要直接打印字符而非ASCII值
// 使用int类型可以直接打印,因为它包含了字符编码
printf("Character: %c\n", ascii_value);
return 0;
}
```
当你运行这段程序,它会打印出 'A' 的ASCII值(65)以及字符本身。
ascll码表c语言怎么转换
在C语言中,ASCII码(American Standard Code for Information Interchange,美国信息交换标准代码)是一种字符编码系统,将每个字符映射到一个唯一的整数值。如果你需要在C语言中转换ASCII码,通常会涉及到以下几个步骤:
1. **获取输入**:首先,你需要读取一个字符或者其对应的整数值作为ASCII码。
2. **转换**:使用`int`类型存储ASCII值。例如,如果你想把字符`'A'`转换成ASCII码,可以这样做:
```c
char ch = 'A';
int ascii_value = (int)ch; // 字符转ASCII码
```
或者如果你已经有整数表示的ASCII值:
```c
int ascii_int = 65; // ASCII值举例
char ch = (char)ascii_int; // ASCII码转字符
```
3. **显示结果**:如果需要打印出来,可以直接输出字符或使用`printf`函数。
注意,ASCII码只包含7位二进制,所以最高位通常都是0,如果需要处理扩展ASCII或其他更复杂的编码,可能需要额外的操作库支持。
相关推荐
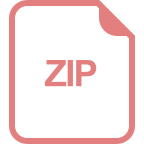
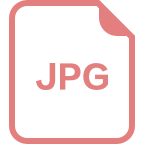
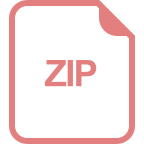






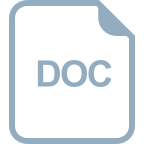
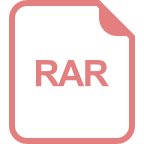
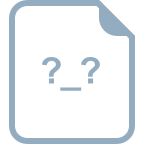
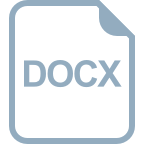


