php发送微信消息通知
时间: 2024-01-29 15:00:50 浏览: 157
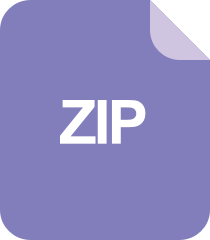
php发送短信
要使用PHP发送微信消息通知,可以使用微信提供的公众号接口或者企业微信接口。
1. 使用公众号接口:
首先需要获取到公众号的access_token,可以通过调用微信提供的API获取。然后使用该access_token来发送消息通知。
示例代码如下:
```php
<?php
// 获取access_token
$appid = 'your_appid';
$secret = 'your_secret';
$apiUrl = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={$appid}&secret={$secret}";
$result = json_decode(file_get_contents($apiUrl), true);
$access_token = $result["access_token"];
// 发送消息
$msg = "Hello, World!";
$openid = 'your_openid';
$apiUrl = "https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token={$access_token}";
$data = array(
'touser' => $openid,
'msgtype' => 'text',
'text' => array(
'content' => $msg
)
);
$options = array(
'http' => array(
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => json_encode($data)
)
);
$context = stream_context_create($options);
$result = file_get_contents($apiUrl, false, $context);
```
以上代码中的`your_appid`、`your_secret`、`your_openid`分别替换成你的公众号的AppID、AppSecret和要发送的用户的openid。
2. 使用企业微信接口:
首先需要在企业微信后台创建一个应用,并获取到应用的access_token。然后使用该access_token来发送消息通知。
示例代码如下:
```php
<?php
// 获取access_token
$corpid = 'your_corpid';
$corpsecret = 'your_corpsecret';
$apiUrl = "https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid={$corpid}&corpsecret={$corpsecret}";
$result = json_decode(file_get_contents($apiUrl), true);
$access_token = $result["access_token"];
// 发送消息
$msg = "Hello, World!";
$userid = 'your_userid';
$apiUrl = "https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token={$access_token}";
$data = array(
'touser' => $userid,
'msgtype' => 'text',
'text' => array(
'content' => $msg
)
);
$options = array(
'http' => array(
'header' => "Content-type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => json_encode($data)
)
);
$context = stream_context_create($options);
$result = file_get_contents($apiUrl, false, $context);
```
以上代码中的`your_corpid`、`your_corpsecret`、`your_userid`分别替换成你的企业微信的corpid、corpsecret和要发送的用户的userid。
阅读全文
相关推荐
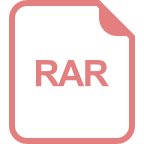
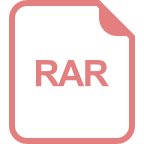
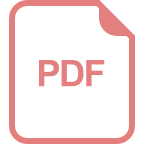
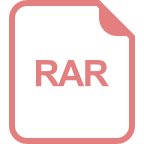
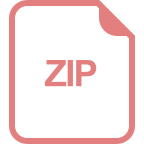
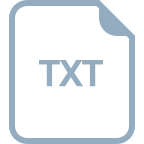
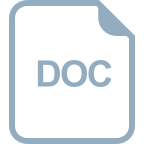
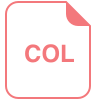
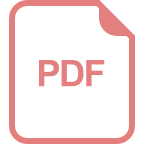
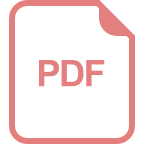
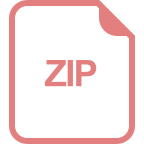
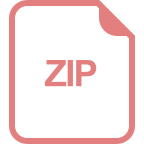
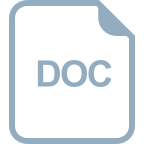
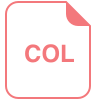

