c语言自定义二维数组
时间: 2024-06-17 17:02:15 浏览: 186
在C语言中,我们可以通过自定义二维数组来表示一个二维矩阵。自定义二维数组的定义方式为:类型名 数组名[行数][列数]。其中,类型名表示数组中元素的数据类型,数组名表示自定义的数组名称,行数和列数分别表示该数组的行数和列数。
例如,我们可以定义一个int类型的二维数组arr,行数为3,列数为4,代码如下:
```
int arr;
```
当然,在定义二维数组时,我们也可以直接初始化数组元素,例如:
```
int arr = {{1, 2, 3}, {4, 5, 6}};
```
这样就定义了一个2行3列的二维数组,其中第1行为{1, 2, 3},第2行为{4, 5, 6}。
相关问题
c语言自定义二维数组data利用双层循环遍历二维数组中各个元素,并输出元素值。
可以使用以下代码实现:
```
int data[3][4] = {{1,2,3,4}, {5,6,7,8}, {9,10,11,12}};
for(int i=0; i<3; i++){
for(int j=0; j<4; j++){
printf("%d ", data[i][j]);
}
printf("\n");
}
```
输出结果为:
```
1 2 3 4
5 6 7 8
9 10 11 12
```
二维数组的定义和初始化自定义二维数组data(数组元素行列值和类型自己选择),利用双层循环遍历二维数组中各个元素,并输出元素值
二维数组是一个表格形式的数据结构,可以理解为数组的数组。在C语言中可以使用以下方式定义和初始化一个自定义二维数组data:
```c
int data[2][3] = { {1, 2, 3}, {4, 5, 6} };
```
这个二维数组有两行三列,每个元素都是整型数值。我们可以通过双层循环遍历二维数组中的元素,并输出它们的值:
```c
for (int i = 0; i < 2; i++) { // 遍历每一行
for (int j = 0; j < 3; j++) { // 遍历每一列
printf("data[%d][%d] = %d\n", i, j, data[i][j]); // 输出元素值
}
}
```
这个例子中,我们使用了两层for循环。外层循环遍历每一行,内层循环遍历每一列,以此输出二维数组中每个元素的值。
阅读全文
相关推荐
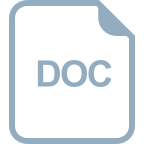
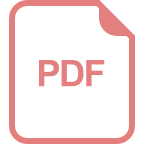
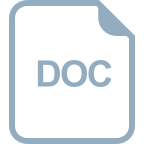
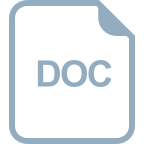
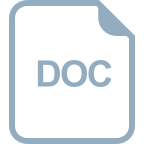
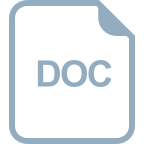







