用c++编写交换两个变量的值的函数,函数参数分别使用原变量、指针变量、引用变量,在主函数调用这三个不同的函数,观察其运行结果的不同。
时间: 2024-09-19 07:02:47 浏览: 24
在 C++ 中,我们可以编写三个函数来交换两个变量的值:一个是通过原始变量传递,另一个是通过指针,第三个是通过引用。以下是三种方法的示例:
1. **直接通过原始变量** (non-recommended due to value semantics)
```cpp
void swapWithoutPointers(int a, int b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swapWithoutPointers(x, y);
// 主函数中看不到x和y的交换效果,因为它们实际上是副本
cout << "x = " << x << ", y = " << y << endl; // 输出:x = 5, y = 10
return 0;
}
```
2. **通过指针** (recommended for efficiency and direct manipulation of memory)
```cpp
void swapByPointer(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
swapByPointer(&x, &y); // 使用地址(&x)传递变量
cout << "x = " << x << ", y = " << y << endl; // 输出:x = 10, y = 5
}
```
3. **通过引用** (most efficient way, directly modifies the original variables)
```cpp
void swapByReference(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swapByReference(x, y); // 直接操作x和y
cout << "x = " << x << ", y = " << y << endl; // 输出:x = 10, y = 5
}
```
相关推荐
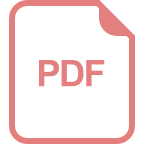
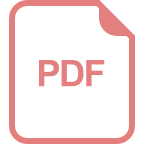
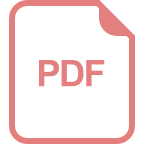














