C语言写一段循环双向链表的代码
时间: 2023-09-17 12:08:21 浏览: 50
#include <stdio.h>
#include <stdlib.h>
// 双向链表结构体
typedef struct doubly_linked_list {
int data; // 数据域
struct doubly_linked_list *prev; // 前驱指针
struct doubly_linked_list *next; // 后继指针
} DoublyLinkedList;
// 创建双向链表
DoublyLinkedList *create_doubly_linked_list(int data) {
DoublyLinkedList *head = (DoublyLinkedList *) malloc(sizeof(DoublyLinkedList));
head->data = data;
head->prev = NULL;
head->next = NULL;
return head;
}
// 在双向链表尾部插入元素
DoublyLinkedList *insert_doubly_linked_list_tail(DoublyLinkedList *head, int data) {
DoublyLinkedList *new_node = (DoublyLinkedList *) malloc(sizeof(DoublyLinkedList));
new_node->data = data;
new_node->next = NULL;
DoublyLinkedList *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_node;
new_node->prev = p;
return head;
}
// 在双向链表头部插入元素
DoublyLinkedList *insert_doubly_linked_list_head(DoublyLinkedList *head, int data) {
DoublyLinkedList *new_node = (DoublyLinkedList *) malloc(sizeof(DoublyLinkedList));
new_node->data = data;
new_node->prev = NULL;
new_node->next = head;
head->prev = new_node;
head = new_node;
return head;
}
// 删除双向链表指定元素
DoublyLinkedList *delete_doubly_linked_list_node(DoublyLinkedList *head, int data) {
DoublyLinkedList *p = head;
while (p != NULL) {
if (p->data == data) {
if (p == head) {
head = p->next;
head->prev = NULL;
} else if (p->next == NULL) {
p->prev->next = NULL;
} else {
p->prev->next = p->next;
p->next->prev = p->prev;
}
free(p);
break;
}
p = p->next;
}
return head;
}
// 输出双向链表
void print_doubly_linked_list(DoublyLinkedList *head) {
DoublyLinkedList *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
DoublyLinkedList *head = create_doubly_linked_list(1);
head = insert_doubly_linked_list_tail(head, 2);
head = insert_doubly_linked_list_tail(head, 3);
head = insert_doubly_linked_list_head(head, 0);
head = delete_doubly_linked_list_node(head, 2);
print_doubly_linked_list(head);
return 0;
}
相关推荐
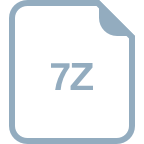
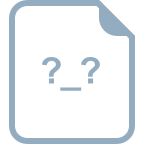
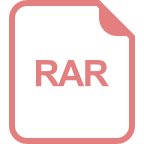














