Feign 每个方法单独设置超时时间
时间: 2023-08-07 19:02:57 浏览: 52
可以通过 Feign 的 `Request.Options` 对象来设置每个方法的超时时间,具体实现可参考以下代码:
```java
public interface MyFeignClient {
@RequestLine("GET /users/{id}")
@Headers("Content-Type: application/json")
User getUserById(@Param("id") Long id, Request.Options options);
}
```
在调用 `getUserById` 方法时,可以通过传入一个 `Request.Options` 对象来设置该方法的超时时间,例如:
```java
MyFeignClient client = Feign.builder()
.target(MyFeignClient.class, "http://localhost:8080");
User user = client.getUserById(123L, new Request.Options(5000, 10000)); // 设置超时时间为 5 秒和 10 秒
```
这样就可以实现每个方法设置不同的超时时间了。
相关问题
FeignClient 每个接口超时时间单独设置
可以使用@FeignClient注解中的configuration属性,指定一个Feign的配置类,然后在该配置类中自定义Feign的超时时间配置。具体实现可以参考以下代码:
```java
@FeignClient(name = "example-service", configuration = ExampleFeignConfiguration.class)
public interface ExampleFeignClient {
@RequestMapping(value = "/example", method = RequestMethod.GET)
String getExampleData();
}
@Configuration
public class ExampleFeignConfiguration {
@Bean
public Request.Options options() {
return new Request.Options(5000, 10000); // 设置连接超时和读取超时时间
}
}
```
在上述代码中,我们自定义了一个Feign的配置类ExampleFeignConfiguration,在该类中通过@Bean注解创建了一个Request.Options对象,并指定了连接超时和读取超时时间。然后在@FeignClient注解中指定了该配置类,从而实现了每个接口超时时间单独设置的效果。
Feign 配置式 设置 每个接口里面每个类的 超时时间
你可以通过在Feign接口的方法上使用`@FeignClient`注解来配置超时时间。具体步骤如下:
1. 在Feign接口上使用`@FeignClient`注解,并设置`configuration`属性为自定义的Feign配置类,例如:
```
@FeignClient(name = "example", configuration = FeignConfig.class)
public interface ExampleFeignClient {
// ...
}
```
2. 创建自定义的Feign配置类,并在其中设置超时时间。例如:
```
@Configuration
public class FeignConfig {
@Bean
public Request.Options options() {
return new Request.Options(5000, 5000);
}
}
```
上面的代码表示设置连接超时时间和读取超时时间都为5秒。
3. 在Feign接口的方法上使用`@Override`注解,并调用超时时间配置方法。例如:
```
@FeignClient(name = "example", configuration = FeignConfig.class)
public interface ExampleFeignClient {
@Override
@RequestMapping(method = RequestMethod.GET, value = "/example")
@Headers("Content-Type: application/json")
ExampleResponse getExample(@RequestParam("id") String id);
}
```
上面的代码表示调用`getExample`方法时,会使用配置类`FeignConfig`中设置的超时时间。
相关推荐
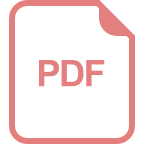
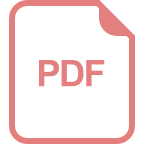
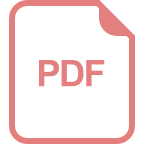












