get Pre-whitening signal by python
时间: 2023-05-24 11:06:27 浏览: 74
Pre-whitening is a technique used in signal processing to remove the spectral correlation of a signal, thus making it easier to analyze or model. Here is an example of how to pre-whiten a signal using Python and the NumPy library.
First, let's import the necessary libraries:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import lfilter, butter
```
Next, let's generate a simple signal consisting of two sinusoids with different frequencies and amplitudes:
```python
fs = 1000 # Sampling rate in Hz
t = np.arange(0, 1, 1/fs) # Time vector from 0 to 1 second
n = len(t) # Number of samples
f1 = 50 # First sinusoid frequency in Hz
f2 = 200 # Second sinusoid frequency in Hz
A1 = 1 # First sinusoid amplitude
A2 = 0.5 # Second sinusoid amplitude
x = A1*np.sin(2*np.pi*f1*t) + A2*np.sin(2*np.pi*f2*t) # Signal
```
We can plot the signal to visualize it:
```python
plt.plot(t, x)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.show()
```

Now we can pre-whiten the signal using a first-order Butterworth high-pass filter with a cutoff frequency of 10 Hz. This will remove the low-frequency components of the signal and leave us with a white noise signal:
```python
f_cutoff = 10 # Cutoff frequency in Hz
b, a = butter(1, f_cutoff/(fs/2), btype='highpass') # High-pass filter coefficients
x_filt = lfilter(b, a, x) # Apply filter to signal
```
We can plot the filtered signal to visualize it:
```python
plt.plot(t, x_filt)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.show()
```

As you can see, the pre-whitened signal has a flat spectral density, which means that its power is uniformly distributed across all frequencies. This makes it easier to analyze or model the signal without being biased by its spectral correlation.
相关推荐
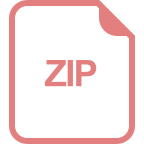
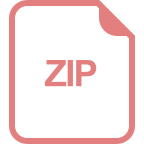
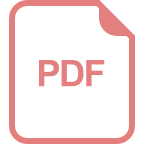














