用qt写一段代码实现对于Socket通讯中客户端发送不同命令的问题,您可以考虑使用多线程来解决。在一个线程中,发送一个命令并等待服务器返回结果,如果需要再发送另一个命令,可以开启一个新的线程来处理。这样可以避免因为等待服务器响应而导致程序无响应或者闪退的问题。
时间: 2023-11-27 16:54:04 浏览: 29
好的,下面是一个基于Qt的多线程实现的Socket通讯客户端代码示例:
```cpp
#include <QtNetwork>
#include <QThread>
class ClientThread : public QThread
{
Q_OBJECT
public:
ClientThread(QObject *parent = nullptr);
~ClientThread();
void setHost(const QString &host);
void setPort(quint16 port);
void setCommand(const QString &command);
QString getResult() const;
protected:
void run() override;
private:
QString m_host;
quint16 m_port;
QString m_command;
QString m_result;
};
ClientThread::ClientThread(QObject *parent) :
QThread(parent)
{
}
ClientThread::~ClientThread()
{
}
void ClientThread::setHost(const QString &host)
{
m_host = host;
}
void ClientThread::setPort(quint16 port)
{
m_port = port;
}
void ClientThread::setCommand(const QString &command)
{
m_command = command;
}
QString ClientThread::getResult() const
{
return m_result;
}
void ClientThread::run()
{
QTcpSocket socket;
socket.connectToHost(m_host, m_port);
if (socket.waitForConnected()) {
socket.write(m_command.toUtf8());
socket.waitForBytesWritten();
if (socket.waitForReadyRead()) {
m_result = socket.readAll();
}
}
}
// 在需要发送不同命令的地方,可以这样使用多线程:
ClientThread *thread = new ClientThread(this);
thread->setHost("127.0.0.1");
thread->setPort(1234);
thread->setCommand("command1");
thread->start();
// 等待线程执行结束并获取结果
thread->wait();
QString result1 = thread->getResult();
// 再次使用线程发送不同命令
thread->setCommand("command2");
thread->start();
thread->wait();
QString result2 = thread->getResult();
```
在这个示例代码中,我们使用了一个继承自 QThread 的 ClientThread 类来处理 Socket 通讯。在主线程中,我们可以实例化多个 ClientThread 对象来处理不同的命令请求。每个 ClientThread 对象都有自己的 host、port、command 和 result 属性。当我们需要发送不同的命令时,只需要创建一个新的 ClientThread 对象并设置相应的属性即可。在执行过程中,每个线程都会独立地连接到服务器并发送自己的命令,并等待服务器返回结果。这样可以避免因为等待服务器响应而导致程序无响应或者闪退的问题。
需要注意的是,在使用多线程时,我们需要确保线程安全性。在这个示例代码中,我们使用了线程本地存储的方式来保证每个线程都有自己的属性,避免了线程之间的竞争。同时,在主线程中,我们使用了 thread->wait() 的方式来等待线程执行结束,并获取线程的执行结果。这样可以确保多个线程之间不会相互干扰。
相关推荐
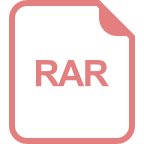
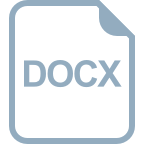
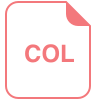













