java通过connection查询mysql
时间: 2023-09-08 21:07:44 浏览: 80
要通过 Java 中的 Connection 对象查询 MySQL 数据库,可以按照以下步骤进行操作:
1. 导入 MySQL 驱动程序包
在 Java 代码中,需要先导入 MySQL 驱动程序包,以便能够连接和操作 MySQL 数据库。可以在 Maven 项目中添加以下依赖项:
```xml
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
```
2. 建立数据库连接
在 Java 代码中,可以通过 Connection 对象建立与 MySQL 数据库的连接。可以使用以下代码:
```java
import java.sql.*;
public class MySQLConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
Connection conn = null;
try {
conn = DriverManager.getConnection(url, user, password);
System.out.println("Connected to the database");
} catch (SQLException e) {
System.out.println(e.getMessage());
} finally {
try {
if (conn != null) {
conn.close();
System.out.println("Disconnected from the database");
}
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
}
```
在上述代码中,url 是 MySQL 数据库的连接地址,user 和 password 分别是数据库的用户名和密码。在建立连接时,需要使用 DriverManager 类的 getConnection() 方法。
3. 执行 SQL 查询语句
在建立数据库连接之后,可以通过 Connection 对象执行 SQL 查询语句。例如,可以使用以下代码查询表中的数据:
```java
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM mytable");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
System.out.println("ID: " + id + ", Name: " + name);
}
} catch (SQLException e) {
System.out.println(e.getMessage());
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
```
在上述代码中,使用 Statement 对象执行 SQL 查询语句,并使用 ResultSet 对象获取查询结果。在查询结果中,使用 getInt() 和 getString() 方法获取相应的数据。
以上就是通过 Java 中的 Connection 对象查询 MySQL 数据库的基本步骤。
阅读全文
相关推荐
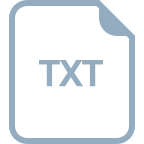
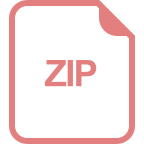
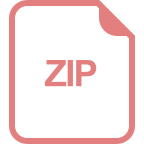
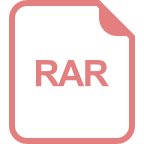
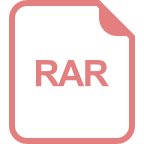
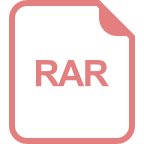
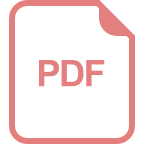
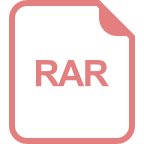
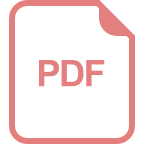
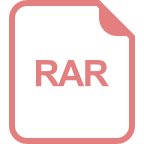
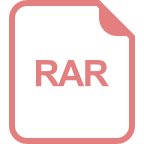
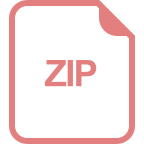
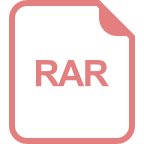
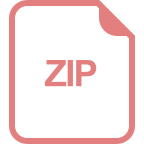
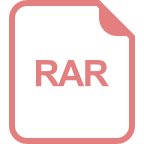
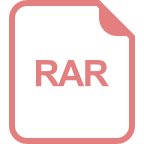
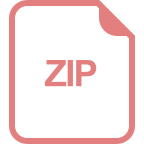