File file = new File("./src/main/java/io/ReadStringDemo.java"); FileInputStream fis = new FileInputStream(file);
时间: 2024-05-27 14:10:10 浏览: 15
这段代码创建了一个 `File` 对象,该对象指向当前项目路径下的 `ReadStringDemo.java` 文件;然后使用 `FileInputStream` 类创建了一个文件输入流对象 `fis`,用于读取该文件的内容。这里需要注意的是,如果文件不存在或无法读取,将会抛出异常。另外,使用完 `fis` 后,应该及时关闭它以释放资源。
相关问题
java.lang.NoClassDefFoundError: jxl/WorkbookSettings
这个错误通常是由于缺少jxl库文件或者类路径不正确导致的。你可以尝试以下几个步骤来解决这个问题:
1. 确认你已经正确地添加了jxl库文件。你可以在项目的构建路径中添加jxl.jar文件,或者将jxl.jar文件复制到项目的lib目录下。
2. 确认你的类路径正确。你可以在命令行中使用java -cp命令来指定类路径,或者在Eclipse中设置项目的类路径。
3. 确认你的jxl库文件版本正确。如果你的代码使用的是旧版本的jxl库文件,而你安装的是新版本的jxl库文件,那么就会出现这个错误。你可以尝试使用旧版本的jxl库文件来解决这个问题。
以下是一个可能的解决方案:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import jxl.Workbook;
import jxl.read.biff.BiffException;
public class ReadExcel {
public static void main(String[] args) {
try {
File file = new File("/mnt/sdcard/Pictures/MyPictures/测试数据.xls");
FileInputStream fis = new FileInputStream(file);
Workbook book = Workbook.getWorkbook(fis);
int num_sheet = book.getNumberOfSheets();
System.out.println(String.valueOf(num_sheet));
book.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
} catch (BiffException e) {
e.printStackTrace();
}
}
}
```
用java帮我写出调用 https://api.mch.weixin.qq.com/v3/certificates接口的方法
好的,以下是Java代码示例,用于调用微信支付V3的证书接口:
```java
import java.io.*;
import java.net.*;
import java.security.KeyStore;
import java.security.PrivateKey;
import java.security.Signature;
import java.security.cert.X509Certificate;
import java.util.Base64;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import javax.net.ssl.*;
import org.apache.commons.io.IOUtils;
public class WeChatPayV3CertApi {
private static final String CERT_FILE = "apiclient_cert.p12"; // 商户证书文件名
private static final String CERT_PASSWORD = "商户证书密码"; // 商户证书密码
private static final String MCH_ID = "商户号"; // 商户号
private static final String API_V3_KEY = "微信支付V3密钥"; // 微信支付V3密钥
private static final String API_V3_CERT_SERIAL_NO = "微信支付V3证书序列号"; // 微信支付V3证书序列号
private static final String API_V3_CERT_PRIVATE_KEY = "微信支付V3证书私钥"; // 微信支付V3证书私钥
private static final String API_HOST = "api.mch.weixin.qq.com";
public static void main(String[] args) {
String result = requestWeChatPayV3Cert();
System.out.println(result);
}
public static String requestWeChatPayV3Cert() {
try {
URL url = new URL("https://" + API_HOST + "/v3/certificates");
HttpsURLConnection conn = (HttpsURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
conn.setReadTimeout(5000);
conn.setRequestProperty("Host", API_HOST);
conn.setRequestProperty("Accept", "application/json");
conn.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36");
conn.setRequestProperty("Authorization", getAuthorizationHeader(url, "GET", ""));
if (conn.getResponseCode() == 200) {
String result = IOUtils.toString(conn.getInputStream(), "UTF-8");
conn.disconnect();
return result;
} else {
String result = IOUtils.toString(conn.getErrorStream(), "UTF-8");
conn.disconnect();
return result;
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
private static String getAuthorizationHeader(URL url, String method, String body) throws Exception {
String nonceStr = Long.toString(new Date().getTime());
String timestamp = Long.toString(new Date().getTime() / 1000);
String message = getMessage(nonceStr, timestamp, url, method, body);
String signature = getSignature(API_V3_CERT_PRIVATE_KEY, message);
Map<String, String> map = new HashMap<String, String>();
map.put("mchid", MCH_ID);
map.put("nonce_str", nonceStr);
map.put("timestamp", timestamp);
map.put("serial_no", API_V3_CERT_SERIAL_NO);
map.put("signature", signature);
StringBuilder sb = new StringBuilder();
for (Map.Entry<String, String> entry : map.entrySet()) {
sb.append(entry.getKey());
sb.append("=\"");
sb.append(entry.getValue());
sb.append("\", ");
}
sb.deleteCharAt(sb.length() - 2);
return "WECHATPAY2-SHA256-RSA2048 " + sb.toString();
}
private static String getMessage(String nonceStr, String timestamp, URL url, String method, String body) {
StringBuilder sb = new StringBuilder();
sb.append(method.toUpperCase());
sb.append('\n');
sb.append(url.getPath());
sb.append('\n');
sb.append(url.getHost().toLowerCase());
sb.append('\n');
sb.append(getQueryString(url));
sb.append('\n');
sb.append("nonce_str=");
sb.append(nonceStr);
sb.append("&");
sb.append("timestamp=");
sb.append(timestamp);
sb.append('\n');
sb.append(body);
return sb.toString();
}
private static String getQueryString(URL url) {
String query = url.getQuery();
if (query == null) {
return "";
} else {
return query;
}
}
private static String getSignature(String privateKeyStr, String message) throws Exception {
byte[] messageBytes = message.getBytes("UTF-8");
byte[] privateKeyBytes = Base64.getDecoder().decode(privateKeyStr);
KeyStore keystore = KeyStore.getInstance("PKCS12");
ByteArrayInputStream bis = new ByteArrayInputStream(privateKeyBytes);
keystore.load(bis, CERT_PASSWORD.toCharArray());
bis.close();
PrivateKey privateKey = (PrivateKey) keystore.getKey("apiclient", CERT_PASSWORD.toCharArray());
X509Certificate certificate = (X509Certificate) keystore.getCertificate("apiclient");
Signature sign = Signature.getInstance("SHA256withRSA");
sign.initSign(privateKey);
sign.update(messageBytes);
byte[] signatureBytes = sign.sign();
return Base64.getEncoder().encodeToString(signatureBytes);
}
private static SSLSocketFactory getSSLFactory() throws Exception {
char[] password = API_V3_KEY.toCharArray();
KeyStore ks = KeyStore.getInstance("PKCS12");
FileInputStream fis = new FileInputStream(CERT_FILE);
ks.load(fis, password);
fis.close();
KeyManagerFactory kmf = KeyManagerFactory.getInstance("SunX509");
kmf.init(ks, password);
TrustManagerFactory tmf = TrustManagerFactory.getInstance("SunX509");
tmf.init(ks);
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(kmf.getKeyManagers(), tmf.getTrustManagers(), null);
return sslContext.getSocketFactory();
}
}
```
请注意,要运行此代码,您需要将商户证书文件apiclient_cert.p12放在当前目录中,并将CERT_PASSWORD替换为您的商户证书密码。
此代码将输出调用微信支付V3的证书接口的响应结果。
相关推荐
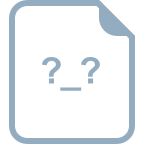












