请用面向对象方法,实现数学上的复数类(数据成员由实部和虚部),要有构造函数,运算符+,-,《的重载 Complex c0; Complex c1(2.3, 4.6); Complex c2 = c1; c0 = 2.2 + c1; cout << c0 << endl;
时间: 2024-05-27 22:13:36 浏览: 92
#include<iostream>
using namespace std;
class Complex{
private:
double real; //实部
double imag; //虚部
public:
Complex():real(0), imag(0){} //默认构造函数
Complex(double r, double i):real(r), imag(i){} //带参数的构造函数
Complex(const Complex& c){real=c.real; imag=c.imag;} //复制构造函数
Complex operator+(const Complex& c) const{ //加法运算符重载
Complex res(real+c.real, imag+c.imag);
return res;
}
Complex operator-(const Complex& c) const{ //减法运算符重载
Complex res(real-c.real, imag-c.imag);
return res;
}
Complex operator*(const Complex& c) const{ //乘法运算符重载
Complex res(real*c.real-imag*c.imag, real*c.imag+imag*c.real);
return res;
}
Complex operator/(const Complex& c) const{ //除法运算符重载
double denominator = c.real*c.real + c.imag*c.imag;
Complex res((real*c.real+imag*c.imag)/denominator, (imag*c.real-real*c.imag)/denominator);
return res;
}
Complex& operator=(const Complex& c){ //赋值运算符重载
if(this == &c) //自我赋值
return *this;
real = c.real;
imag = c.imag;
return *this;
}
bool operator<(const Complex& c) const{ //小于运算符重载
double mod1 = real*real + imag*imag;
double mod2 = c.real*c.real + c.imag*c.imag;
return mod1 < mod2;
}
friend ostream& operator<<(ostream& os, const Complex& c); //输出运算符重载
};
ostream& operator<<(ostream& os, const Complex& c){ //输出运算符重载实现
os << "(" << c.real << "," << c.imag << "i)";
return os;
}
int main(){
Complex c0; //默认构造函数
Complex c1(2.3, 4.6); //带参数的构造函数
Complex c2 = c1; //复制构造函数
c0 = 2.2*c1; //赋值运算符重载
cout << c0 << endl; //输出运算符重载
return 0;
}
阅读全文
相关推荐




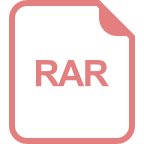


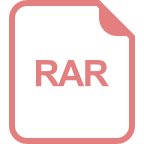
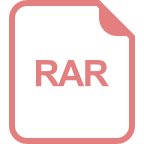

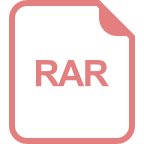
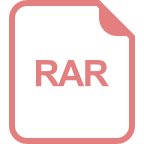




