用c#写一个简单的计算器窗口
时间: 2024-09-23 07:16:49 浏览: 22
在C#中创建一个简单的图形用户界面(GUI)计算器窗口,可以使用Windows Forms库。以下是一个基本步骤的示例:
1. **设置项目**:
首先,打开Visual Studio,选择“新建”->“项目”,然后选择“Windows Forms App (.NET Framework)”模板。
2. **设计用户界面**:
在Form1.cs designer上,你可以添加以下控件:
- 数字键、运算符按钮(如数字0-9,+,-,*,/等)
- 温馨提示:可以使用`NumericUpDown`控件作为数字键,`Button`控件用于运算符。
```csharp
private void InitializeComponent()
{
// 添加数字键
for (int i = 0; i <= 9; i++)
{
Button digitButton = new Button();
digitButton.Text = i.ToString();
digitButton.Click += DigitButtonClick;
Controls.Add(digitButton);
}
// 添加运算符按钮...
}
```
3. **事件处理**:
对于每个按钮点击事件,需要捕获用户的输入并更新当前的计算结果。例如,对于数字键:
```csharp
private decimal currentResult = 0;
private void DigitButtonClick(object sender, EventArgs e)
{
Button button = (Button)sender;
if (button.Name == "Digit0") currentResult *= 10;
else
{
decimal value = Convert.ToDecimal(button.Text);
currentResult += value;
}
DisplayResult(currentResult);
}
private void OperationButtonClick(object sender, EventArgs e)
{
Button operatorButton = (Button)sender;
PerformOperation(operatorButton.Tag as string);
}
```
4. **显示结果**:
可能需要一个文本框来显示当前的结果,比如`DisplayResult()`方法:
```csharp
private void DisplayResult(decimal result)
{
ResultTextBox.Text = result.ToString();
}
```
5. **处理运算操作**:
`PerformOperation(string op)`函数会根据用户点击的运算符进行相应的数学操作。
6. **完整窗口初始化和关闭**:
初始化窗体和处理关闭事件:
```csharp
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 初始化其他控件,如运算符按钮
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
// 保存状态或清理资源
}
}
```
相关推荐
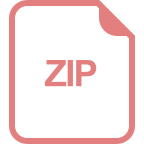
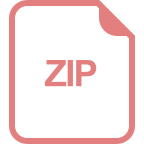
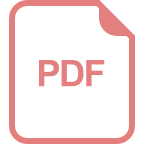











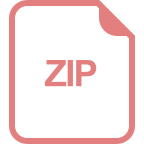
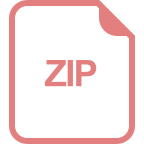
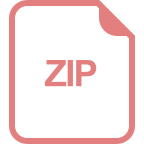