使用vue3封装wangeditor富文本组件
时间: 2023-08-02 19:06:29 浏览: 191
好的,我可以为您介绍一下使用Vue3封装WangEditor富文本组件的步骤。
首先,我们需要安装WangEditor和Vue3,可以通过以下命令进行安装:
```bash
npm install wangeditor@latest
npm install vue@next
```
接着,我们可以在Vue3的组件中使用WangEditor。下面是一个简单的示例:
```vue
<template>
<div ref="editorElem"></div>
</template>
<script>
import WangEditor from 'wangeditor'
export default {
mounted() {
const editor = new WangEditor(this.$refs.editorElem)
editor.create()
}
}
</script>
```
在上面的代码中,我们通过import导入了WangEditor,并在mounted钩子函数中创建了一个新的编辑器实例。注意,我们需要在组件的模板中添加一个ref属性,用于引用编辑器的DOM元素。
如果您需要进一步封装WangEditor组件,可以考虑将其封装为一个Vue组件,以便在其他地方重复使用。下面是一个简单的示例:
```vue
<template>
<div :id="editorId"></div>
</template>
<script>
import WangEditor from 'wangeditor'
export default {
props: {
value: String,
placeholder: String,
height: {
type: String,
default: '300px'
}
},
data() {
return {
editorId: `editor-${Math.random().toString(36).substr(2, 9)}`,
editor: null
}
},
mounted() {
this.editor = new WangEditor(`#${this.editorId}`)
this.editor.config.height = this.height
this.editor.config.placeholder = this.placeholder
this.editor.config.onchange = this.handleChange
this.editor.create()
this.editor.txt.html(this.value)
},
methods: {
handleChange() {
this.$emit('input', this.editor.txt.html())
}
},
beforeUnmount() {
this.editor.destroy()
}
}
</script>
```
在上面的代码中,我们定义了一个WangEditor组件,并通过props接收了一些参数,包括组件的初始值、占位符和高度等。在mounted钩子函数中,我们创建了一个新的编辑器实例,并通过config属性设置了一些编辑器的配置项,包括高度、占位符和内容变化时的回调函数等。我们还通过handleChange方法监听了编辑器内容的变化,并通过$emit方法向父组件发送了一个input事件,以便在父组件中更新组件的绑定值。最后,我们在beforeUnmount钩子函数中销毁了编辑器实例,以避免内存泄漏。
使用时,您可以像使用其他自定义组件一样,在Vue3的模板中引用WangEditor组件,并通过v-model指令绑定组件的值:
```vue
<template>
<div>
<wangeditor v-model="content" placeholder="请输入内容" height="500px" />
<div>{{ content }}</div>
</div>
</template>
<script>
import WangEditor from '@/components/WangEditor.vue'
export default {
components: {
WangEditor
},
data() {
return {
content: ''
}
}
}
</script>
```
在上面的代码中,我们通过import导入了WangEditor组件,并在模板中引用了该组件。我们还通过v-model指令绑定了组件的值,以便在父组件中获取和更新该值。
阅读全文
相关推荐
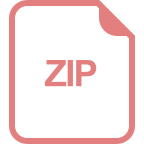
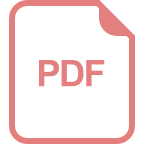

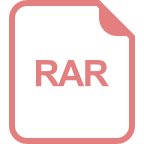
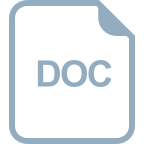


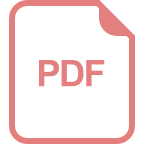
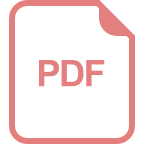
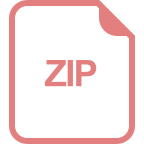
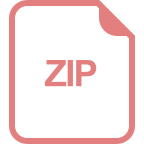
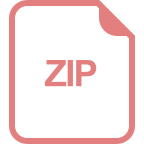





