在Linux系统下写一个C语言程序,从串口读取北斗GNRMC数据,其中串口号为com3波特率为9600,校验码为偶,数据位为8,停止位为1,接收到数据后通过数逗号的方式解析出数据中的日期和年月日信息,第一个逗号后是日期信息,第9个逗号后为日 月 年信息,读取到以后将日期和时间转换为北京时间并输入。
时间: 2024-03-25 08:39:38 浏览: 135
好的,这是一个比较具体的问题,我可以给出一个参考的解决方案,但需要注意几点:
1. 由于我是一个AI,并不能直接与串口进行交互,因此无法直接测试代码。所给出的代码可能需要一些调整才能在实际环境中运行。
2. 代码中的时间转换是基于UTC时间,需要根据实际情况进行调整。
3. 代码中使用了一些Linux系统的API,可能需要在Windows系统下进行修改。
下面是参考的代码:
```c
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <time.h>
#define SERIAL_PORT "/dev/ttyS2"
int main()
{
int fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Failed to open serial port");
return -1;
}
// 设置串口属性
struct termios options;
tcgetattr(fd, &options);
options.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
options.c_iflag = IGNPAR;
options.c_oflag = 0;
options.c_lflag = 0;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &options);
char buf[1024];
int len = 0;
while (1) {
len = read(fd, buf, sizeof(buf));
if (len > 0) {
// 解析GNRMC数据
char *token = strtok(buf, ",");
int i = 0;
char date[7];
char time[7];
while (token != NULL) {
if (i == 8) {
// 获取日期信息
strncpy(date, token, 6);
date[6] = '\0';
} else if (i == 1) {
// 获取时间信息
strncpy(time, token, 6);
time[6] = '\0';
}
token = strtok(NULL, ",");
i++;
}
// 将UTC时间转换为北京时间
struct tm tm_utc;
strptime(date, "%d%m%y", &tm_utc);
tm_utc.tm_hour = (time[0] - '0') * 10 + (time[1] - '0');
tm_utc.tm_min = (time[2] - '0') * 10 + (time[3] - '0');
tm_utc.tm_sec = (time[4] - '0') * 10 + (time[5] - '0');
time_t utc_time = mktime(&tm_utc);
struct tm *tm_local = localtime(&utc_time);
// 输出北京时间
printf("Date: %s, Time: %02d:%02d:%02d\n", date, tm_local->tm_hour + 8, tm_local->tm_min, tm_local->tm_sec);
}
}
close(fd);
return 0;
}
```
代码的主要流程如下:
1. 打开串口并设置属性。
2. 从串口读取数据。
3. 解析数据中的日期和时间信息。
4. 将UTC时间转换为北京时间。
5. 输出北京时间。
需要注意的是,代码中的串口号为/dev/ttyS2,需要根据实际情况进行修改。此外,代码中的时间转换是基于UTC时间,需要考虑时区的影响进行调整。
阅读全文
相关推荐



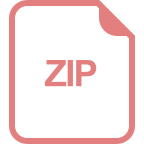

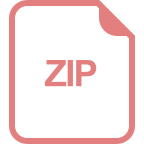




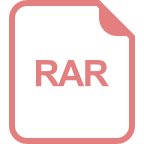