python 提取特定字段
时间: 2023-07-18 13:37:40 浏览: 68
如果你想从一个字符串或者文本中提取特定的字段,可以考虑使用正则表达式。
下面是一个简单的例子,假设你想从一个字符串中提取出所有的数字:
```python
import re
text = "The price of the product is $123.45"
pattern = '\d+'
result = re.findall(pattern, text)
print(result)
```
运行上面的代码,输出结果为:
```
['123', '45']
```
正则表达式 `\d+` 匹配所有的数字,`re.findall()` 函数返回所有匹配的结果。
如果你想提取更复杂的字段,你可以根据实际情况编写更复杂的正则表达式。
相关问题
Python的list 如何提取指定内容字段
要提取列表中的特定字段,可以使用列表推导式。假设你有一个包含字典的列表,每个字典都有多个键值对。你可以使用以下代码来提取特定字段:
```python
original_list = [
{'name': 'Alice', 'age': 25, 'city': 'New York'},
{'name': 'Bob', 'age': 30, 'city': 'San Francisco'},
{'name': 'Charlie', 'age': 35, 'city': 'Los Angeles'}
]
# 提取每个字典中的'name'和'age'字段
new_list = [{'name': d['name'], 'age': d['age']} for d in original_list]
print(new_list)
# Output: [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
```
在上面的代码中,我们使用列表推导式来创建一个新的列表,其中每个元素都是一个字典,只包含原始列表中每个字典的'name'和'age'字段。你可以根据需要修改字典中的键名称和要提取的字段。
实现python对于json的特定数据提取
在Python中,您可以使用`json`模块来解析和提取特定数据。下面是一个示例代码,演示了如何从JSON数据中提取特定字段:
```python
import json
# 假设以下是一个包含JSON数据的字符串
json_str = '''
{
"name": "John",
"age": 30,
"city": "New York",
"skills": ["Python", "Java", "C++"]
}
'''
# 解析JSON数据
data = json.loads(json_str)
# 提取特定字段
name = data["name"]
age = data["age"]
city = data["city"]
# 打印提取的结果
print("Name:", name)
print("Age:", age)
print("City:", city)
```
在上面的代码中,我们首先将包含JSON数据的字符串`json_str`传递给`json.loads()`函数进行解析,返回一个Python字典对象。然后,我们可以通过字典的键来提取特定字段的值。
在这个示例中,我们提取了"name"、"age"和"city"这三个字段,并将它们分别存储在对应的变量中。最后,我们打印出这些提取的结果。
请注意,如果JSON数据的结构更加复杂,可能需要使用更多的嵌套键来访问特定的数据。您可以根据实际情况来调整代码来提取所需的数据。
相关推荐
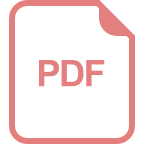












