pychram写,一个有对话框窗口的文件夹目录下所有文件夹和文件的名称关键字替换软件
时间: 2023-12-03 10:46:36 浏览: 107
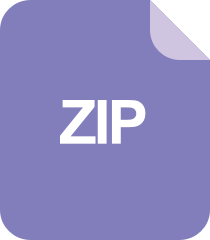
基于Python实现的人脸识别系统【100010666】
下面是一个简单的实现,使用了PyQt5作为GUI框架:
```python
import os
from PyQt5.QtWidgets import QApplication, QWidget, QFileDialog, QVBoxLayout, QHBoxLayout, QLabel, QLineEdit, QPushButton, QTextEdit
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("文件夹名称关键字替换软件")
self.setGeometry(200, 200, 800, 600)
# UI组件
self.label_input = QLabel("请选择要修改的文件夹:")
self.edit_input = QLineEdit()
self.button_input = QPushButton("选择文件夹")
self.label_keyword = QLabel("请输入要替换的关键字:")
self.edit_keyword = QLineEdit()
self.label_replace = QLabel("请输入要替换成的关键字:")
self.edit_replace = QLineEdit()
self.button_start = QPushButton("开始替换")
self.text_output = QTextEdit()
# 布局
self.layout_input = QHBoxLayout()
self.layout_input.addWidget(self.label_input)
self.layout_input.addWidget(self.edit_input)
self.layout_input.addWidget(self.button_input)
self.layout_keyword = QHBoxLayout()
self.layout_keyword.addWidget(self.label_keyword)
self.layout_keyword.addWidget(self.edit_keyword)
self.layout_replace = QHBoxLayout()
self.layout_replace.addWidget(self.label_replace)
self.layout_replace.addWidget(self.edit_replace)
self.layout_button = QHBoxLayout()
self.layout_button.addStretch()
self.layout_button.addWidget(self.button_start)
self.layout_button.addStretch()
self.layout_main = QVBoxLayout()
self.layout_main.addLayout(self.layout_input)
self.layout_main.addLayout(self.layout_keyword)
self.layout_main.addLayout(self.layout_replace)
self.layout_main.addLayout(self.layout_button)
self.layout_main.addWidget(self.text_output)
self.setLayout(self.layout_main)
# 绑定事件
self.button_input.clicked.connect(self.select_folder)
self.button_start.clicked.connect(self.start_replace)
def select_folder(self):
folder_path = QFileDialog.getExistingDirectory(self, "选择文件夹")
self.edit_input.setText(folder_path)
def start_replace(self):
folder_path = self.edit_input.text()
keyword = self.edit_keyword.text()
replace = self.edit_replace.text()
if not os.path.exists(folder_path):
self.text_output.append("文件夹不存在!")
return
for root, dirs, files in os.walk(folder_path):
for name in files + dirs:
if keyword in name:
old_path = os.path.join(root, name)
new_name = name.replace(keyword, replace)
new_path = os.path.join(root, new_name)
os.rename(old_path, new_path)
self.text_output.append(f"已将 {old_path} 修改为 {new_path}")
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
使用该软件时,先选择要修改的文件夹,然后输入要替换的关键字和替换成的关键字,点击“开始替换”按钮即可。替换结果会输出在文本框中。
阅读全文