遍历文件夹下面所有的文件路径 c
时间: 2024-09-11 22:03:24 浏览: 44
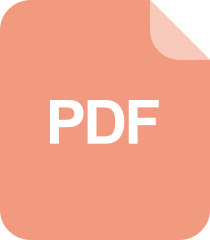
C语言 实现遍历一个文件夹的所有文件
在C++中,可以使用递归的方式遍历文件夹下面所有的文件路径。具体步骤如下:
1. 使用Windows API中的FindFirstFile和FindNextFile函数遍历文件夹下的所有文件和子文件夹。
2. 对于每一个文件,可以使用fstream库中的ifstream对象读取文件内容,并输出文件路径和文件内容。
3. 对于每一个子文件夹,可以递归调用遍历函数,直到遍历完所有文件。
下面是一个简单的示例代码:
```
#include <iostream>
#include <fstream>
#include <windows.h>
using namespace std;
void TraverseFolder(string folderPath) {
WIN32_FIND_DATA findData;
HANDLE hFind = FindFirstFile((folderPath + "\\*").c_str(), &findData);
if (hFind == INVALID_HANDLE_VALUE) {
return;
}
do {
if (findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) {
if (strcmp(findData.cFileName, ".") != 0 && strcmp(findData.cFileName, "..") != 0) {
TraverseFolder(folderPath + "\\" + findData.cFileName);
}
} else {
ifstream file(folderPath + "\\" + findData.cFileName);
if (file.is_open()) {
cout << "File path: " << folderPath + "\\" + findData.cFileName << endl;
string line;
while (getline(file, line)) {
cout << line << endl;
}
file.close();
}
}
} while (FindNextFile(hFind, &findData));
FindClose(hFind);
}
int main() {
TraverseFolder("C:\\test");
return 0;
}
```
阅读全文
相关推荐
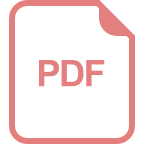
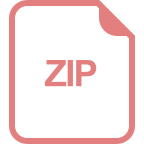
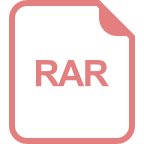
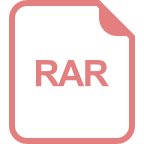
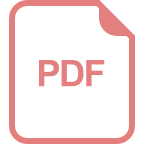
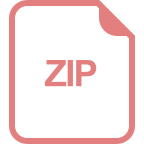
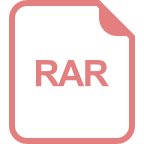
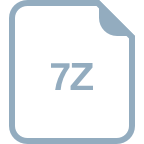
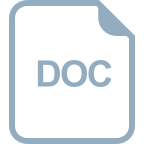







