android studio搜索框功能
时间: 2023-05-01 08:00:06 浏览: 80
Android Studio中可以使用搜索框功能来查找文件、类、方法等。你可以在菜单栏中选择"Edit" -> "Find" -> "Find in Path" 或者使用快捷键 "Ctrl+Shift+F" 来打开搜索框。在搜索框中输入关键字,点击 "Find" 按钮即可查找到相关内容。
相关问题
android studio搜索框功能实现
要实现Android Studio中的搜索框功能,可以使用SearchView控件和OnQueryTextListener接口。首先,在布局文件中添加SearchView控件,并设置相应的属性,如图标、提示文本等。然后,在Activity中获取SearchView的实例,并通过setOnQueryTextListener()方法设置监听器。在监听器的回调方法中,可以获取用户输入的文字,并进行相应的处理。
在代码中,可以使用以下步骤实现搜索框功能:
1. 在布局文件中添加SearchView控件,并设置相应属性,如iconifiedByDefault和queryHint。
2. 在Activity中获取SearchView的实例。
3. 使用setOnQueryTextListener()方法为SearchView设置监听器。
4. 在监听器的onQueryTextSubmit()方法中处理用户提交的搜索文字。
5. 在监听器的onQueryTextChange()方法中处理用户输入的实时搜索文字。
以下是一个示例代码:
```java
// 在布局文件中添加SearchView控件
<androidx.appcompat.widget.SearchView
android:id="@+id/searchView"
android:layout_width="200dp"
android:layout_height="wrap_content"
app:iconifiedByDefault="false"
app:queryHint="请输入搜索内容" />
// 在Activity中获取SearchView的实例,并设置监听器
SearchView searchView = findViewById(R.id.searchView);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// 处理用户提交的搜索文字
Log.i("TAG", "搜索的文字:" + query);
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
// 处理用户输入的实时搜索文字
Log.i("TAG", "输入的文字:" + newText);
return false;
}
});
```
常用属性:
- iconifiedByDefault:是否锁定搜索框为展开状态,false表示锁定(放大镜在搜索框外)
android studio 搜索框
在 Android Studio 中,可以使用搜索框来快速查找项目中的文件、类、方法等。搜索框通常位于 Android Studio 界面的右上角。你可以通过以下步骤打开搜索框:
1. 打开 Android Studio。
2. 在 Android Studio 界面右上角找到搜索框。
3. 点击搜索框或者使用快捷键 Ctrl+Shift+F(Windows 和 Linux)或者 Command+Shift+F(Mac)来打开搜索框。
4. 在搜索框中输入关键字,例如文件名、类名、方法名等。
5. 按下 Enter 键或者点击搜索框右侧的搜索按钮,Android Studio 将会搜索项目中所有匹配关键字的文件、类、方法等,并将结果显示在搜索框下方的搜索结果窗口中。
搜索框是 Android Studio 中非常常用的功能之一,它可以帮助你快速定位并查找项目中的相关内容,提高开发效率。
相关推荐
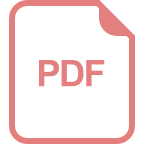
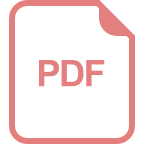
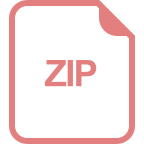












