帮我写一个完整的串口收发数据C语言代码
时间: 2024-04-29 07:24:14 浏览: 108
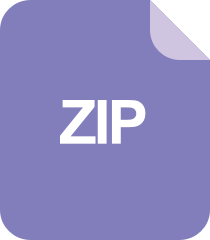
串口数据收发,串口数据收发代码,C/C++

以下是一个简单的串口收发数据的C语言代码,可以用于Windows和Linux系统。
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int set_serial(int fd, int baudrate, int databits, int stopbits, char parity)
{
struct termios options;
tcgetattr(fd, &options);
// 设置波特率
switch (baudrate)
{
case 2400:
cfsetispeed(&options, B2400);
cfsetospeed(&options, B2400);
break;
case 4800:
cfsetispeed(&options, B4800);
cfsetospeed(&options, B4800);
break;
case 9600:
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
break;
case 19200:
cfsetispeed(&options, B19200);
cfsetospeed(&options, B19200);
break;
case 38400:
cfsetispeed(&options, B38400);
cfsetospeed(&options, B38400);
break;
case 57600:
cfsetispeed(&options, B57600);
cfsetospeed(&options, B57600);
break;
case 115200:
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
break;
default:
fprintf(stderr, "Unsupported baudrate.\n");
return -1;
}
// 设置数据位
options.c_cflag &= ~CSIZE;
switch (databits)
{
case 5:
options.c_cflag |= CS5;
break;
case 6:
options.c_cflag |= CS6;
break;
case 7:
options.c_cflag |= CS7;
break;
case 8:
options.c_cflag |= CS8;
break;
default:
fprintf(stderr, "Unsupported data size.\n");
return -1;
}
// 设置停止位
switch (stopbits)
{
case 1:
options.c_cflag &= ~CSTOPB;
break;
case 2:
options.c_cflag |= CSTOPB;
break;
default:
fprintf(stderr, "Unsupported stop bits.\n");
return -1;
}
// 设置奇偶校验位
switch (parity)
{
case 'n':
case 'N':
options.c_cflag &= ~PARENB;
options.c_iflag &= ~INPCK;
break;
case 'o':
case 'O':
options.c_cflag |= (PARODD | PARENB);
options.c_iflag |= INPCK;
break;
case 'e':
case 'E':
options.c_cflag |= PARENB;
options.c_cflag &= ~PARODD;
options.c_iflag |= INPCK;
break;
default:
fprintf(stderr, "Unsupported parity.\n");
return -1;
}
// 设置输入输出流控制
options.c_cflag &= ~CRTSCTS;
// 设置非规范模式
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
// 设置字符间隔和超时时间
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
// 清空串口缓冲区
tcflush(fd, TCIFLUSH);
// 设置串口属性
if (tcsetattr(fd, TCSANOW, &options) != 0)
{
fprintf(stderr, "Set serial attributes error.\n");
return -1;
}
return 0;
}
int open_serial(const char* portname)
{
int fd = open(portname, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0)
{
perror("Open serial port error");
return -1;
}
// 设置串口为阻塞模式
fcntl(fd, F_SETFL, 0);
return fd;
}
int close_serial(int fd)
{
close(fd);
return 0;
}
int send_data(int fd, const char* data, int len)
{
int n = write(fd, data, len);
if (n < 0)
{
perror("Write data error");
return -1;
}
return n;
}
int recv_data(int fd, char* data, int len)
{
int n = read(fd, data, len);
if (n < 0)
{
perror("Read data error");
return -1;
}
return n;
}
int main()
{
int fd = open_serial("/dev/ttyUSB0");
if (fd < 0) return -1;
if (set_serial(fd, 115200, 8, 1, 'N') < 0)
{
close_serial(fd);
return -1;
}
char sendbuf[] = "Hello, world!";
if (send_data(fd, sendbuf, strlen(sendbuf)) < 0)
{
close_serial(fd);
return -1;
}
char recvbuf[1024] = {0};
if (recv_data(fd, recvbuf, sizeof(recvbuf)) < 0)
{
close_serial(fd);
return -1;
}
printf("Received: %s\n", recvbuf);
close_serial(fd);
return 0;
}
```
这个代码中,`set_serial()`函数用于设置串口的属性,包括波特率、数据位、停止位、奇偶校验位等等。`open_serial()`函数用于打开串口设备,`close_serial()`函数用于关闭串口设备。`send_data()`和`recv_data()`函数分别用于发送和接收数据。
在`main()`函数中,我们首先打开串口设备`/dev/ttyUSB0`,然后设置串口属性,接着发送一个字符串,最后接收一个字符串并打印出来。你可以根据自己的需要修改这个代码,例如更改串口设备名称、波特率、发送和接收的数据等等。
阅读全文
相关推荐
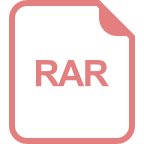
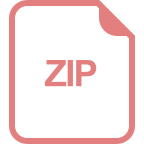
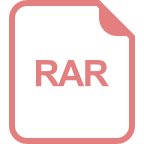
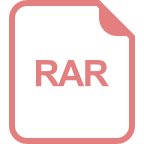
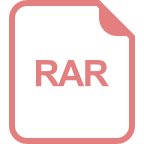
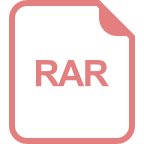
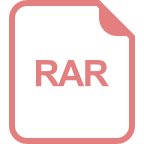
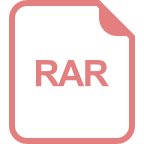
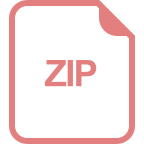
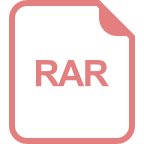
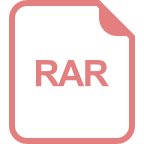
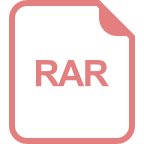
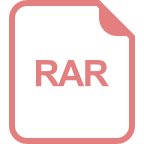
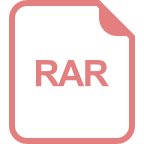
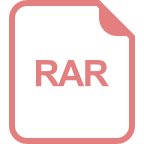