针对上面的问题,若是有多张 JPG图片和多个json文件如何增强,请给出Python代码
时间: 2024-02-05 17:12:28 浏览: 69
针对这个问题,需要先确定增强的具体方式是什么,不同的增强方式可能需要不同的代码实现。这里提供一个常见的数据增强方式——随机旋转,并给出Python代码实现。
假设有多张JPG图片和对应的JSON标注文件,我们可以使用Python中的Pillow库和OpenCV库来进行图像处理和数据增强。
首先,需要安装Pillow和OpenCV库,可以使用以下命令进行安装:
```
pip install Pillow
pip install opencv-python
```
然后,可以使用以下代码实现随机旋转的数据增强:
```python
import os
import json
import random
import cv2
from PIL import Image
def augment_data(img_dir, ann_dir):
# 获取图片文件和标注文件列表
img_files = os.listdir(img_dir)
ann_files = os.listdir(ann_dir)
# 遍历每个图片文件和对应的标注文件
for img_file, ann_file in zip(img_files, ann_files):
# 读取图片和标注数据
img_path = os.path.join(img_dir, img_file)
img = cv2.imread(img_path)
ann_path = os.path.join(ann_dir, ann_file)
with open(ann_path, 'r') as f:
ann_data = json.load(f)
# 随机旋转图片和标注数据
angle = random.uniform(-10, 10) # 随机角度
h, w = img.shape[:2]
center = (w // 2, h // 2)
M = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated_img = cv2.warpAffine(img, M, (w, h))
for obj in ann_data['objects']:
points = obj['points']
points = [(p[0], p[1]) for p in points]
rotated_points = []
for p in points:
x, y = p
x_new = (x - center[0]) * M[0][0] + (y - center[1]) * M[0][1] + center[0]
y_new = (x - center[0]) * M[1][0] + (y - center[1]) * M[1][1] + center[1]
rotated_points.append([x_new, y_new])
obj['points'] = rotated_points
# 保存旋转后的图片和标注数据
rotated_img_path = os.path.join(img_dir, f"rotated_{angle:.2f}_{img_file}")
cv2.imwrite(rotated_img_path, rotated_img)
rotated_ann_path = os.path.join(ann_dir, f"rotated_{angle:.2f}_{ann_file}")
with open(rotated_ann_path, 'w') as f:
json.dump(ann_data, f)
if __name__ == '__main__':
img_dir = 'path/to/img/dir'
ann_dir = 'path/to/ann/dir'
augment_data(img_dir, ann_dir)
```
上述代码中,`img_dir`和`ann_dir`分别表示存储图片文件和标注文件的目录。具体实现中,我们遍历每个图片文件和对应的标注文件,使用`cv2.getRotationMatrix2D()`函数随机生成旋转矩阵,并将其应用到图片上,同时也需要对标注数据进行旋转。最后保存旋转后的图片和标注数据。
需要注意的是,上述代码中只实现了对单个标注框的旋转,如果标注文件中包含多个标注框,则需要对每个标注框进行类似的旋转操作。
阅读全文
相关推荐
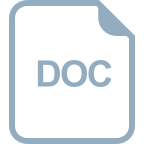
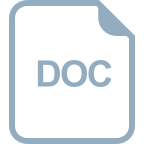
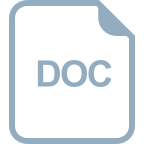





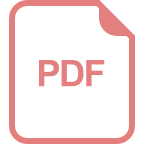
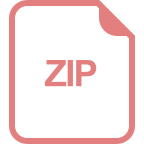








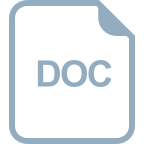